本帖最后由 小梁老师 于 2022-8-12 02:18 编辑
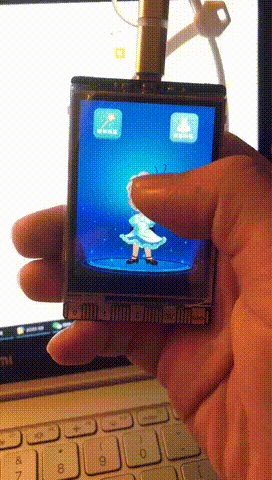
【效果预览】
一、学习内容
运用行空板触摸屏、AB键、加速度传感器设计一款变装小游戏。
1.在触摸屏上,单击头发切换发型、单击衣服切换服装、单击鞋子切换款式
2.在AB键上,单击A键“成套变装”、单击B键“随机变装”3.在加速度传感器上,实现摇一摇恢复初始化状态。
二、学习对象
适合有Python基础五年级以上学生学习
三、学习目标
1.了解pinpong库的加载,熟悉pgzrun(pygame zero)库的使用
2.掌握pgzrun初始化列表、角色和位置的方法
3.学会draw()函数的用法、理解自定义函数格式
4.学会运用if条件语句触发事件
四、学习重难点
1.重点:行空板板载按键和传感器调用自定义函数
2.难点:鼠标事件调用自定义函数
五、学习过程
1.加载第三方库,此项目使用了板载AB按键和加速度传感器,所以需要加载pinpong库;还使用了pygame zero等功能实现互动,需要加载pgzrun、random等相关库。
- # 加载第三方库
- from pinpong.extension.unihiker import *
- from pinpong.board import *
- import pygame
- import pgzrun
- import random
- import time
复制代码
2.初始化pinpong库和游戏中的多个角色
- # 初始化pinpong库
- Board().begin()
- # 设置屏幕大小
- WIDTH = 240
- HEIGHT = 320
- # 创建女孩角色并设置角色位置
- girl = Actor("co")
- girl.pos = 120, 203
- # 创建发型列表、发型角色,设置角色位置
- hair_style = ['hair_1', 'hair_2', 'hair_3', 'hair_4']
- hair = Actor("hair_1")
- hair.pos = 126, 176
- # 创建服装列表、服装角色,设置角色位置
- dress_style = ['dress_1', 'dress_2', 'dress_3', 'dress_4']
- dress = Actor("dress_1")
- dress.pos = 124, 238
- # 创建鞋子列表、鞋子角色,设置角色位置
- shoes_style = ['shoes_1', 'shoes_2', 'shoes_3', 'shoes_4']
- shoes = Actor("shoes_1")
- shoes.pos = 124, 280
- # 创建随机换装按钮,设置角色位置
- button1 = Actor("rand")
- button1.pos = 50, 40
- # 创建成套换装按钮,设置角色位置
- button2 = Actor("match")
- button2.pos = 187, 40
复制代码
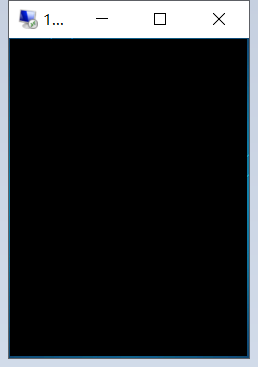
3.使用draw( )函数显示角色和背景
- def draw():
- screen.surface = pygame.display.set_mode((WIDTH, HEIGHT), pygame.FULLSCREEN)
- # 设置背景
- screen.blit('background', (0, 0))
- # 显示所有角色
- girl.draw()
- hair.draw()
- dress.draw()
- shoes.draw()
- button1.draw()
- button2.draw()
复制代码
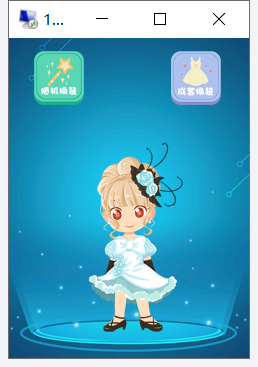
4.定义changestyle()实现变装造型函数,利用列表切换造型。
- # 变换造型函数
- def changestyle(obj, styleList):
- style = styleList.index(obj.image)
- if (style < 3):
- obj.image = styleList[style + 1]
- else:
- obj.image = styleList[0]
复制代码
5.定义change_all()函数,利用random随机库实现成套变装。
- # 成套变装函数
- def change_all():
- suit = random.randint(0, 3)
- dress.image = dress_style[suit]
- hair.image = hair_style[suit]
- shoes.image = shoes_style[suit]
复制代码
6.定义rand_change()函数,利用random随机库实现随机变装。
- # 随机变装函数
- def rand_change():
- dress.image = random.choice(dress_style)
- hair.image = random.choice(hair_style)
- shoes.image = random.choice(shoes_style)
复制代码
7.添加鼠标事件,实现单击发型、服装、鞋子进行造型切换,单击屏幕按钮实现随机变装或成套变装。
- # 当鼠标按下,让鼠标点击的角色变换造型
- def on_mouse_down(pos):
- if dress.collidepoint(pos):
- changestyle(dress, dress_style)
- elif hair.collidepoint(pos):
- changestyle(hair, hair_style)
- elif shoes.collidepoint(pos):
- changestyle(shoes, shoes_style)
- elif button1.collidepoint(pos):
- rand_change()
- elif button2.collidepoint(pos):
- change_all()
复制代码
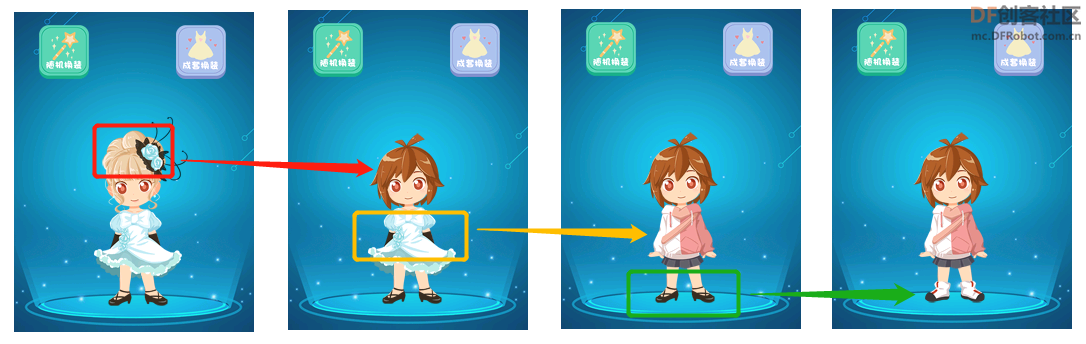
8.使用update()函数更新造型,实现单击板载AB键和摇一摇变装。
- def update():
- # 单击行空板A键调用成套变装函数
- if keyboard.a:
- change_all()
- time.sleep(1)
- # 单击行空板B键调用随机变装函数
- if keyboard.b:
- rand_change()
- time.sleep(1)
- # 摇一摇,实现装扮初始化
- if accelerometer.get_strength() > 1.5:
- dress.image = dress_style[0]
- hair.image = hair_style[0]
- shoes.image = shoes_style[0]
-
- pgzrun.go()
复制代码
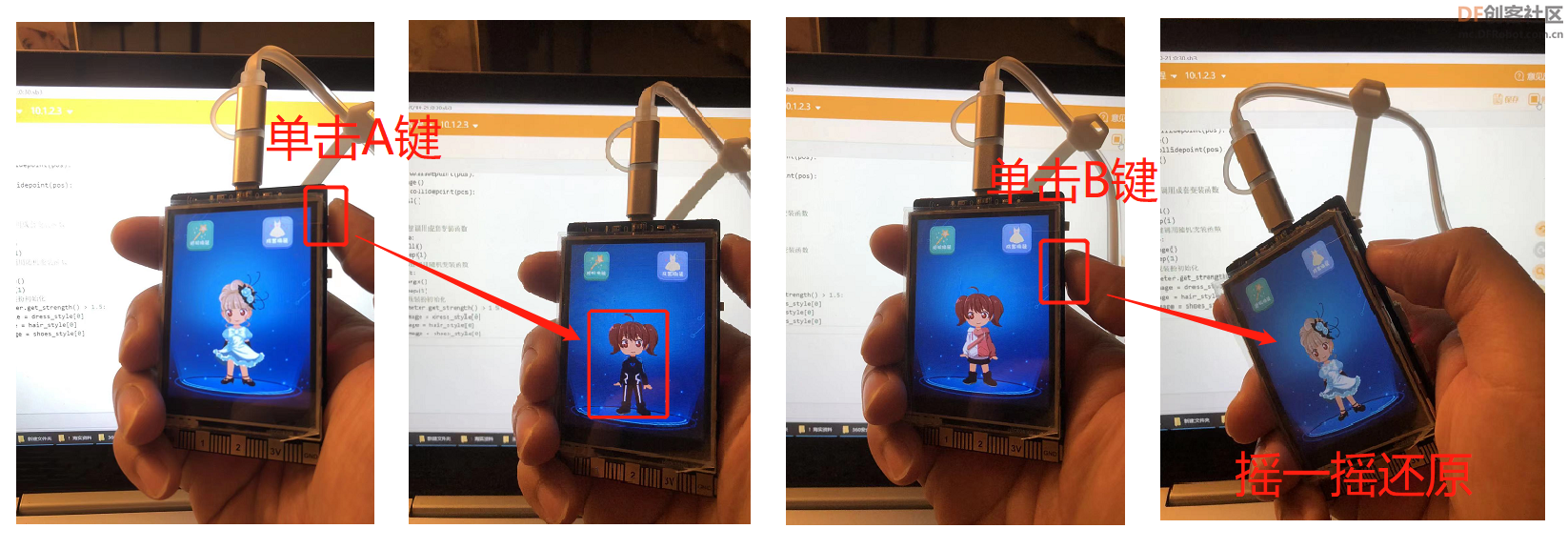
六、总结提升
1.是否可以设计更多玩法,比如结合板载麦克风实现声音控制变装?
2.基于在本项目的思路,设计触屏互动小游戏,比如扫雷、纸牌等。
变装小游戏源码及素材.zip
|