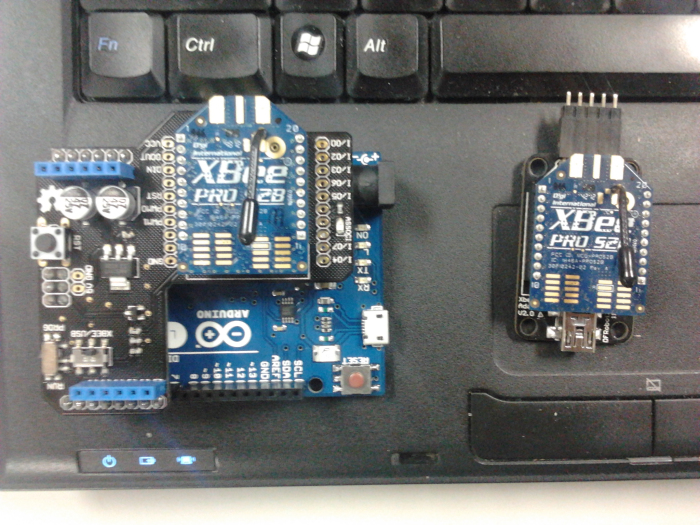
这是我们做的东西的方案 左边的 作为Router 放在移动终端
右边的作为Coordinator 通过适配器连接在PC上
确认设备连接和跳线设置没有问题
现在Router端的associ灯不停闪烁
然后我们写了一个测试程序,烧在Arduino上面
- #include <XBee.h>
- #define disSH 0x0013a200
- #define disSL 0x40a62ddc
- // create the XBee object
- XBee xbee = XBee();
- uint8_t payload[] = { 0, 0 };
- // SH + SL Address of receiving XBee
- XBeeAddress64 addr64 = XBeeAddress64(disSH,disSL);
- ZBTxRequest zbTx = ZBTxRequest(addr64, payload, sizeof(payload));
- ZBTxStatusResponse txStatus = ZBTxStatusResponse();
- int pin5 = 0;
- int statusLed = 13;
- int errorLed = 13;
- void flashLed(int pin, int times, int wait) {
- for (int i = 0; i < times; i++) {
- digitalWrite(pin, HIGH);
- delay(wait);
- digitalWrite(pin, LOW);
- if (i + 1 < times) {
- delay(wait);
- }
- }
- }
- void setup() {
- pinMode(statusLed, OUTPUT);
- pinMode(errorLed, OUTPUT);
- Serial.begin(9600);
- xbee.setSerial(Serial);
- }
- void loop() {
-
- xbee.send(zbTx);
- // flash TX indicator
- flashLed(statusLed, 1, 100);
- // after sending a tx request, we expect a status response
- // wait up to half second for the status response
- if (xbee.readPacket(500)) {
- // got a response!
- // should be a znet tx status
- if (xbee.getResponse().getApiId() == ZB_TX_STATUS_RESPONSE) {
- xbee.getResponse().getZBTxStatusResponse(txStatus);
- // get the delivery status, the fifth byte
- if (txStatus.getDeliveryStatus() == SUCCESS) {
- // success. time to celebrate
- flashLed(statusLed, 5, 50);
- } else {
- // the remote XBee did not receive our packet. is it powered on?
- flashLed(errorLed, 3, 500);
- }
- }
- } else if (xbee.getResponse().isError()) {
- //nss.print("Error reading packet. Error code: ");
- //nss.println(xbee.getResponse().getErrorCode());
- } else {
- // local XBee did not provide a timely TX Status Response -- should not happen
- flashLed(errorLed, 2, 50);
- }
- delay(1000);
- }
复制代码
结果L灯一直在闪烁 flashLed(errorLed, 2, 50); 表示工作不正常。以上为发送测试程序,就是简单的发送一个payload
于是我们又写了一个接收程序- <blockquote>#include <XBee.h>
复制代码
就是简单地 接收到信息
然后我们通过Coordinator端发送广播包,无效,发送单播点对点包,也无效。L一直不亮
Java广播程序如下- /**
- * Copyright (c) 2008 Andrew Rapp. All rights reserved.
- *
- * This file is part of XBee-API.
- *
- * XBee-API is free software: you can redistribute it and/or modify
- * it under the terms of the GNU General Public License as published by
- * the Free Software Foundation, either version 3 of the License, or
- * (at your option) any later version.
- *
- * XBee-API is distributed in the hope that it will be useful,
- * but WITHOUT ANY WARRANTY; without even the implied warranty of
- * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
- * GNU General Public License for more details.
- *
- * You should have received a copy of the GNU General Public License
- * along with XBee-API. If not, see <<a href="http://www.gnu.org/licenses/>." target="_blank">http://www.gnu.org/licenses/>.</a>
- */
-
- package com.rapplogic.xbee.examples.zigbee;
-
- import org.apache.log4j.Logger;
- import org.apache.log4j.PropertyConfigurator;
-
- import com.rapplogic.xbee.api.XBee;
- import com.rapplogic.xbee.api.XBeeAddress64;
- import com.rapplogic.xbee.api.XBeeException;
- import com.rapplogic.xbee.api.zigbee.ZNetTxRequest;
- import com.rapplogic.xbee.util.ByteUtils;
-
- /**
- * @author andrew
- */
- public class BroadcastSenderExample {
-
- private final static Logger log = Logger.getLogger(BroadcastSenderExample.class);
-
- private BroadcastSenderExample() throws XBeeException {
-
- XBee xbee = new XBee();
-
- try {
- // replace with your com port and baud rate. this is the com port of my coordinator
- xbee.open("COM5", 9600);
-
- while (true) {
- // put some arbitrary data in the payload
- int[] payload = ByteUtils.stringToIntArray("the\nquick\nbrown\nfox");
-
- ZNetTxRequest request = new ZNetTxRequest(XBeeAddress64.BROADCAST, payload);
- // make it a broadcast packet
- request.setOption(ZNetTxRequest.Option.BROADCAST);
-
- //log.info("request packet bytes (base 16) " + ByteUtils.toBase16(request.getXBeePacket().getPacket()));
-
- xbee.sendAsynchronous(request);
- // we just assume it was sent. that's just the way it is with broadcast.
- // no transmit status response is sent, so don't bother calling getResponse()
-
- try {
- // wait a bit then send another packet
- Thread.sleep(2000);
- } catch (InterruptedException e) {
- }
- }
- } finally {
- xbee.close();
- }
- }
-
- public static void main(String[] args) throws XBeeException, InterruptedException {
- PropertyConfigurator.configure("log4j.properties");
- new BroadcastSenderExample();
- }
- }
复制代码
使用的是http://code.google.com/p/xbee-api/的最新版本api
两个xbee都在api mode (AP = 2模式下工作)
以下为两个XBee的配置
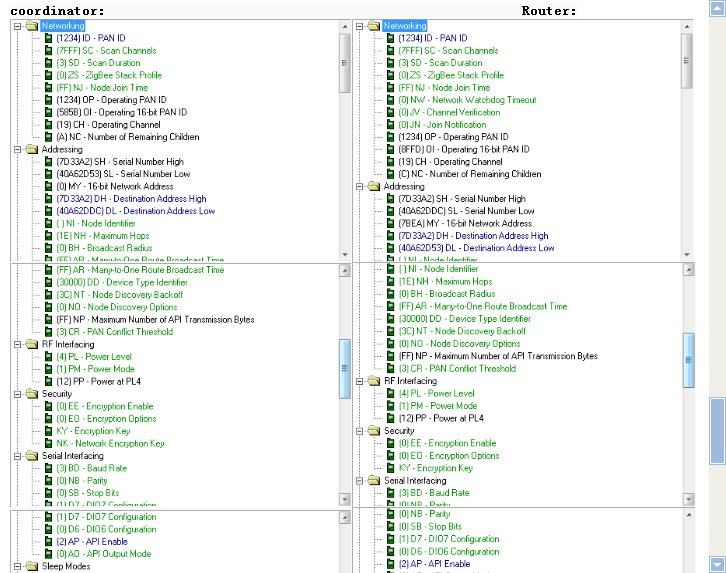
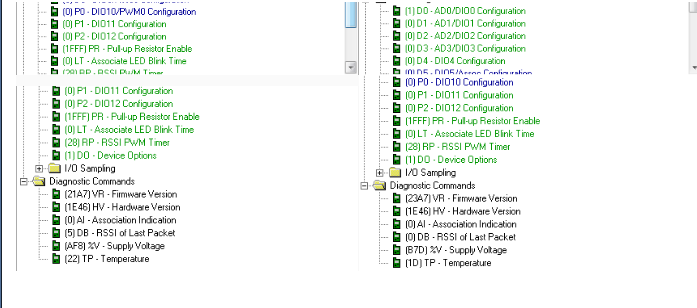
但是如果把两个xbee都放在适配器上 一个发送广播通信 一个接受广播通信 就没有问题 正常工作。
广播接收代码- package com.rapplogic.xbee.examples.zigbee;
-
- import org.apache.log4j.Logger;
- import org.apache.log4j.PropertyConfigurator;
-
- import com.rapplogic.xbee.api.XBee;
- import com.rapplogic.xbee.api.XBeeException;
- import com.rapplogic.xbee.api.XBeeResponse;
-
- /**
- * @author andrew
- */
- public class BroadcastReceiverExample {
-
- private final static Logger log = Logger.getLogger(BroadcastReceiverExample.class);
-
- private BroadcastReceiverExample() throws XBeeException {
-
- XBee xbee = new XBee();
-
- try {
- // replace with your com port and baud rate. this is the com port of my coordinator
- xbee.open("COM5", 9600);
-
- while (true) {
- XBeeResponse response = xbee.getResponse();
- log.info("received response " + response);
- }
- } finally {
- xbee.close();
- }
- }
-
- public static void main(String[] args) throws XBeeException, InterruptedException {
- PropertyConfigurator.configure("log4j.properties");
- new BroadcastReceiverExample();
- }
- }
复制代码
这个是arduino api
http://code.google.com/p/xbee-ar ... l-beta.zip&can=2&q=
麻烦请老板验证下是什么问题 如果确实是api兼容性问题的话 我们就考虑换成不用api的方案 或者是我们这个实现方案有问题,请老板指出,十分感谢。
|