此为全部代码。我只加了一个一元一次功能以及解题步骤功能,具体作者是谁我不知道,这是我以前的作品,直到现在才发。想要增加新代码可以自己加(既然是我搬运的并做了一些小更改那就不需要标明是我的程序了)可以加一个清屏的代码。注释不全是我加的,只有差不多两处我自己写的才是我加的,其他都是原作者
- #include <iostream>
- #include <string>
- #include <cmath>
- using namespace std;
-
- // 快速幂函数,用于计算 a 的 b 次幂
- long long Poww(long long a, long long b) {
- long long ans = 1;
- long long base = a;
- while (b != 0) {
- if (b & 1)
- ans *= base;
- base *= base;
- b >>= 1;
- }
- return ans;
- }
-
- // 阶乘函数,计算 n 的阶乘
- long long f(int n) {
- if (n == 1)
- return 1;
- if (n > 1)
- return n * f(n - 1);
- }
-
- // 计算表达式的值,并输出每一步的计算过程
- double cal(double s, double e, string str);
-
- // 获取一个操作数或括号内表达式的值,并输出计算过程
- double getVal(double &s, double e, string str) {
- if (str[s] == '(' || str[s] == '[' || str[s] == '{') {
- double news, newe, i;
- i = 1;
- news = s + 1;
- while (i) {
- s++;
- if (s == e)
- break;
- if (str[s] == '(' || str[s] == '[' || str[s] == '{')
- i++;
- else if (str[s] == ')' || str[s] == ']' || str[s] == '}')
- i--;
- }
- newe = s - 1;
- s++;
- double result = cal(news, newe, str);
- cout << "计算括号内表达式 " << str.substr(news, newe - news + 1) << " 的值为: " << result << endl;
- return result;
- }
- double val;
- if (str[s] == 's') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = sin(arg);
- cout << "计算 sin(" << arg << ") 的值为: " << val << endl;
- } else if (str[s] == 'c') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = cos(arg);
- cout << "计算 cos(" << arg << ") 的值为: " << val << endl;
- } else if (str[s] == 't') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = tan(arg);
- cout << "计算 tan(" << arg << ") 的值为: " << val << endl;
- } else if (str[s] == 'a') {
- s++;
- if (str[s] == 's') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = asin(arg);
- cout << "计算 asin(" << arg << ") 的值为: " << val << endl;
- } else if (str[s] == 'c') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = acos(arg);
- cout << "计算 acos(" << arg << ") 的值为: " << val << endl;
- } else if (str[s] == 't') {
- s = s + 3;
- double arg = getVal(s, e, str);
- val = atan(arg);
- cout << "计算 atan(" << arg << ") 的值为: " << val << endl;
- }
- } else if (str[s] >= '0' && str[s] <= '9') {
- val = str[s++] - '0';
- while (s < e && str[s] >= '0' && str[s] <= '9') {
- val *= 10;
- val += str[s++] - '0';
- }
- if (str[s] == '!') {
- int n = static_cast<int>(val);
- val = f(n);
- cout << "计算 " << n << "! 的值为: " << val << endl;
- s++;
- }
- if (str[s] == '.') {
- s = s + 1;
- double val2 = str[s++] - '0';
- while (s < e && str[s] >= '0' && str[s] <= '9') {
- val2 *= 10;
- val2 += str[s++] - '0';
- }
- while (val2 >= 1) {
- val2 /= 10;
- }
- val = val + val2;
- cout << "处理小数部分,得到值为: " << val << endl;
- }
- }
- return val;
- }
-
- // 处理乘、除、幂运算,并输出计算过程
- double getVal1(double &s, double e, string str) {
- double val;
- val = getVal(s, e, str);
- while (1) {
- if (s < e && str[s] == '*') {
- double right = getVal(++s, e, str);
- double prev = val;
- val *= right;
- cout << "计算 " << prev << " * " << right << " 的值为: " << val << endl;
- } else if (s < e && str[s] == '/') {
- double right = getVal(++s, e, str);
- double prev = val;
- val /= right;
- cout << "计算 " << prev << " / " << right << " 的值为: " << val << endl;
- } else if (s < e && str[s] == '^') {
- double right = getVal(++s, e, str);
- double prev = val;
- val = Poww(static_cast<long long>(val), static_cast<long long>(right));
- cout << "计算 " << prev << " ^ " << right << " 的值为: " << val << endl;
- } else
- return val;
- }
- }
-
- // 处理加、减运算,并输出计算过程
- double cal(double s, double e, string str) {
- double sum = 0;
- if (str[s] != '-')
- sum = getVal1(s, e, str);
- while (s < e) {
- if (str[s] == '+') {
- double right = getVal1(++s, e, str);
- double prev = sum;
- sum += right;
- cout << "计算 " << prev << " + " << right << " 的值为: " << sum << endl;
- } else if (str[s] == '-') {
- double right = getVal1(++s, e, str);
- double prev = sum;
- sum -= right;
- cout << "计算 " << prev << " - " << right << " 的值为: " << sum << endl;
- }
- }
- return sum;
- }
-
- // 解析方程,将方程分为左右两部分
- void parseEquation(const string& equation, string& left, string& right) {
- size_t equalPos = equation.find('=');
- if (equalPos != string::npos) {
- left = equation.substr(0, equalPos);
- right = equation.substr(equalPos + 1);
- }
- }
-
- // 合并同类项,计算 x 的系数和常数项
- void collectTerms(const string& expr, double& xCoeff, double& constant) {
- double currentCoeff = 1;
- double currentSign = 1;
- double num = 0;
- bool hasX = false;
- for (size_t i = 0; i < expr.length(); ++i) {
- if (isdigit(expr[i])) {
- num = num * 10 + (expr[i] - '0');
- } else if (expr[i] == 'x') {
- hasX = true;
- if (i == 0 || !isdigit(expr[i - 1])) {
- num = 1;
- }
- } else if (expr[i] == '+' || expr[i] == '-') {
- if (hasX) {
- xCoeff += currentSign * currentCoeff * num;
- } else {
- constant += currentSign * num;
- }
- num = 0;
- hasX = false;
- currentSign = (expr[i] == '+') ? 1 : -1;
- currentCoeff = 1;
- }
- }
- if (hasX) {
- xCoeff += currentSign * currentCoeff * num;
- } else {
- constant += currentSign * num;
- }
- }
-
- // 解一元一次方程
- double solveEquation(const string& equation) {
- string left, right;
- parseEquation(equation, left, right);
-
- double leftXCoeff = 0, leftConstant = 0;
- double rightXCoeff = 0, rightConstant = 0;
-
- collectTerms(left, leftXCoeff, leftConstant);
- collectTerms(right, rightXCoeff, rightConstant);
-
- double totalXCoeff = leftXCoeff - rightXCoeff;
- double totalConstant = rightConstant - leftConstant;
-
- if (totalXCoeff == 0) {
- if (totalConstant == 0) {
- cout << "方程有无数解。" << endl;
- } else {
- cout << "方程无解。" << endl;
- }
- return 0;
- }
-
- return totalConstant / totalXCoeff;
- }
- while (cin >> x) {
- if (x.find('x') != string::npos && x.find('=') != string::npos) {
- // 包含未知数 x 且有等号,认为是一元一次方程
- cout << "开始解方程: " << x << endl;
- double solution = solveEquation(x);
- if (solution != 0) {
- cout << "方程的解为: x = " << solution << endl;
- }
- } else {
- // 普通表达式,进行计算并输出步骤
- cout << "开始计算表达式: " << x << endl;
- double result = cal(0, x.length(), x);
- cout << "最终计算结果为: " << result << endl;
- }
- }
- return 0;
- }
复制代码
示范:
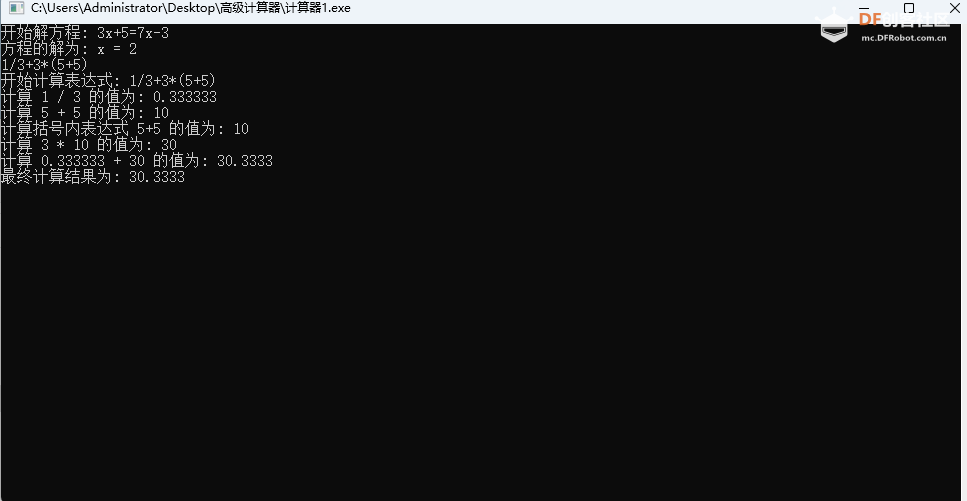
代码缩进风格与上贴一致。
|