本帖最后由 iooops 于 2016-3-13 23:07 编辑
上课笔记!
至于楼主为啥要这么费心还要去学Python?
因为 Python非常适合为树莓派编程。
要在树莓派里面做点啥好玩的交互(引脚的编程一般用Python写)吧?用Python!
要为树莓派做个啥小游戏吧?用Python!
当然用其他语言,如Java也是可以的!
【工具篇】
介绍一个对于初学者而言非常实用的网站:
http://www.codeskulptor.org
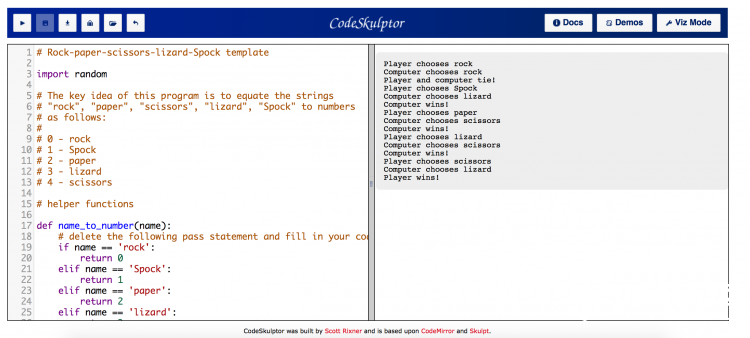
有文件保存(云)、下载、打开、撤销、运行【左】,以及docs(Python的说明教程)、Demos、Viz Mode(这个很神奇,可以亲自试试)【右】。
这个网站是这节课上用到的工具之一。
当然你也可以在shell(比如终端)上运行Python,或者写.py文档并用终端命令执行。
【笔记篇】
下面是第一二周的课程内容(编程语言大都长得差不多)。
http://www.codeskulptor.org/#examples-arithmetic_expressions.py
# 后面的是注释
- 数据类型、强制类型转换、取近似值、int/float运算、运算优先级
- # Arithmetic expressions - numbers, operators, expressions
-
- print 3, -1, 3.14159, -2.8
-
- # numbers - two types, an integer or a decimal number
- # two corresponding data types int() and float()
-
- print type(3), type(3.14159)
- print type(3.0)
-
-
- # we can convert between data types using int() and float()
- # note that int() takes the "whole" part of a decimal number and doesn't round
- # float() applied to integers is boring
-
- print int(3.14159), int(-2.8)
- print float(3), float(-1)
-
-
- # floating point number have around 15 decimal digits of accuracy
- # pi is 3.1415926535897932384626433832795028841971...
- # square root of two is 1.4142135623730950488016887242096980785696...
-
- # approximation of pi, Python displays 12 decimal digits
-
- print 3.1415926535897932384626433832795028841971
-
- # appoximation of square root of two, Python displays 12 decimal digits
-
- print 1.4142135623730950488016887242096980785696
-
- # arithmetic operators
- # + plus addition
- # - minus subtraction
- # * times multiplication
- # / divided by division
- # ** power exponentiation
-
- print 1 + 2, 3 - 4, 5 * 6, 2 ** 5
-
- # Division in Python 2
- # If one operand is a decimal (float), the answer is decimal
-
- print 1.0 / 3, 5.0 / 2.0, -7 / 3.0
-
- # If both operands are ints, the answer is an int (rounded down)
-
- print 1 / 3, 5 / 2, -7 / 3
-
-
- # expressions - number or a binary operator applied to two expressions
- # minus is also a unary operator and can be applied to a single expression
-
- print 1 + 2 * 3, 4.0 - 5.0 / 6.0, 7 * 8 + 9 * 10
-
- # expressions are entered as sequence of numbers and operations
- # how are the number and operators grouped to form expressions?
- # operator precedence - "please excuse my dear aunt sallie" = (), **, *, /, +,-
-
- print 1 * 2 + 3 * 4
- print 2 + 12
-
-
- # always manually group using parentheses when in doubt
-
-
- print 1 * (2 + 3) * 4
- print 1 * 5 * 4
复制代码
variables:
function:
- 注意缩进(function的body部分)
- 有head和body(做computation)两部分
- function可嵌套使用(在一个function的body里使用另一个function)
- 有return值的function返回变量,可赋值
- 无return值的function返回none
  - # compute the area of the triangle
- def triangle_area(base, height): //function - head
- area = (1.0 / 2) * base * height //body starts
- return area //body ends
-
- a1 = triangle_area(3, 8)
- print a1
- a2 = triangle_area(14, 2)
- print a2
-
- # convert fahrenheit to celsius
- def ftoc(fah):
- cel = (5.0 / 9) * (fah -32)
- return cel
-
- # test
- c1 = ftoc(32)
- c2 = ftoc(212)
- print c1, c2
-
- # convert fahrenheit to keivin
- def ftok(fah):
- c = ftoc(fah)
- kelvin = c + 273.15
- return kelvin
-
- # test
- k1 = ftok(32)
- k2 = ftok(212)
- print k1, k2
-
- # prints hellp, world!
- def hello():
- print "Hello, world!"
-
- # test
- hello()
- h = hello()
- print h
复制代码
一个非常实用的算法(求余(%)的妙用!):
有些懒得敲代码了 - - 直接截课程图
如果我们要实现时钟的进位要怎么做呢?
如果用12小时制,8:00 p.m. 到4:00 a.m. 要进位直接用Python写会很麻烦有木有!不然你可以试试?
如果用24小时制,20:00 到 4:00要进位直接用Python写……还是很麻烦有木有……
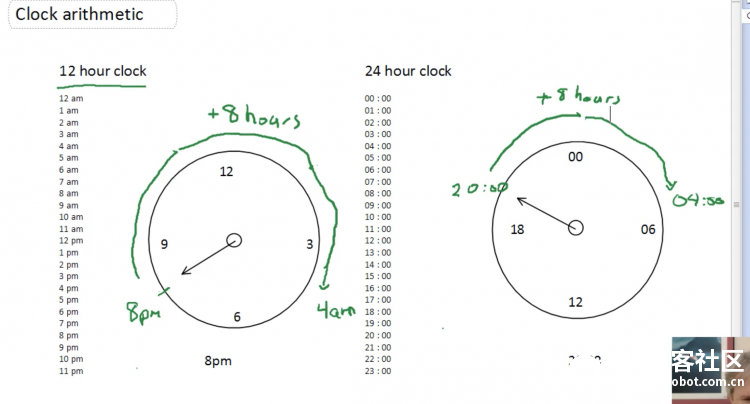
但是!如果我们用了求余的时钟算法,问题就会变得异常简单……
- num = 49
- tens = num // 10 # //是求商数
- ones = num % 10 # %试求余数
- #不知道商数和余数是啥的请翻阅小学一年级课本
复制代码
求余的时钟算法是这样的:
- hour = 20
- shift = 8
- print (hour + shift) % 24 #这就是我们要的4了
复制代码
哈哈哈哈哈哈哈!!有木有觉得这个算法很神奇!!!
其实这个算法用途还蛮广的。
有的时候我们写游戏嘛 = = 我们有个scrren width(比如是1280)还有height的嘛。有个object的width position要超界了(跑到3800去了)咋办?
用这个算法:
- width = 1280
- position = 2
- move = 5
- position = (position + move) % width
- print position
复制代码
当然还有很多其他应用,不一一列举。
下面的例子是讲将number转换为string, 合并输出,就不会两个number之间有空格了。
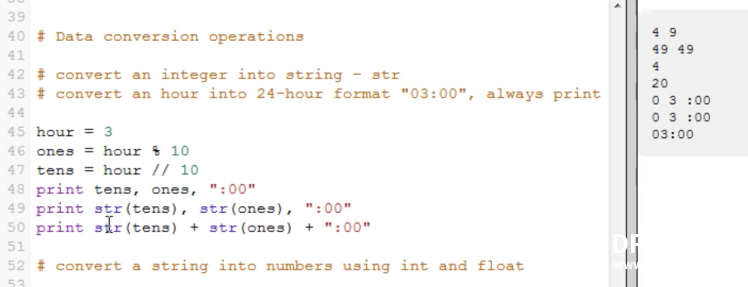
下面的例子是讲bool类型(True/False)之间的逻辑运算(and/or/not):
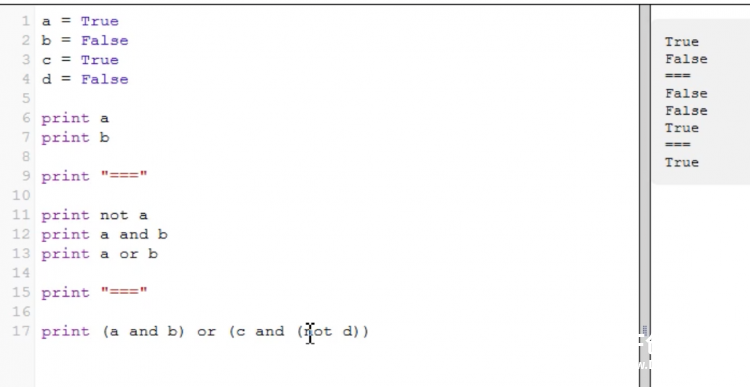
下面是讲comparison operator(+ - * / == !=)的bool运算……再结合逻辑运算:
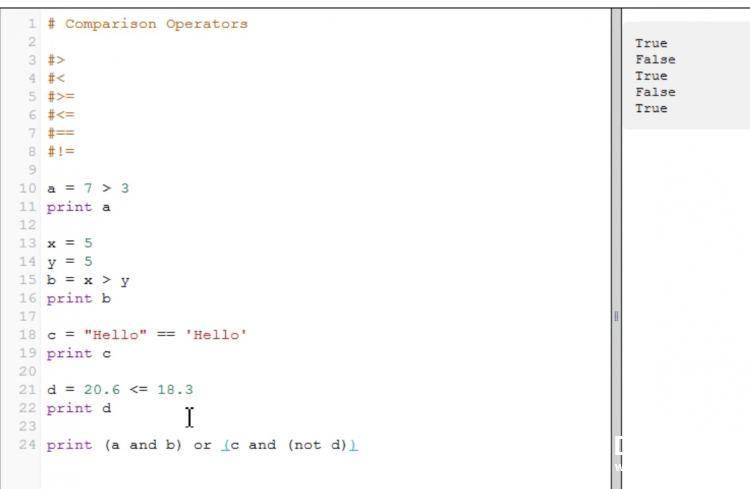
一些细节性的使用要查阅文档:
http://www.codeskulptor.org/docs.html#tabs-Python
这是非常适合于这门课的查阅文档。
以上。两天刷完……还是觉得进度有点慢……
|