本文介绍在树莓派上使用python和qt开发GUI程序,程序功能为显示DS18B20模块的温度曲线。开发环境依然使用之前介绍的PyCharm编写python代码和远程开发,然后使用QtCreator编写QML界面的方式。
1、新建项目1.1、新建工程
打开PyCharm,新建工程tempMonitor,如下:
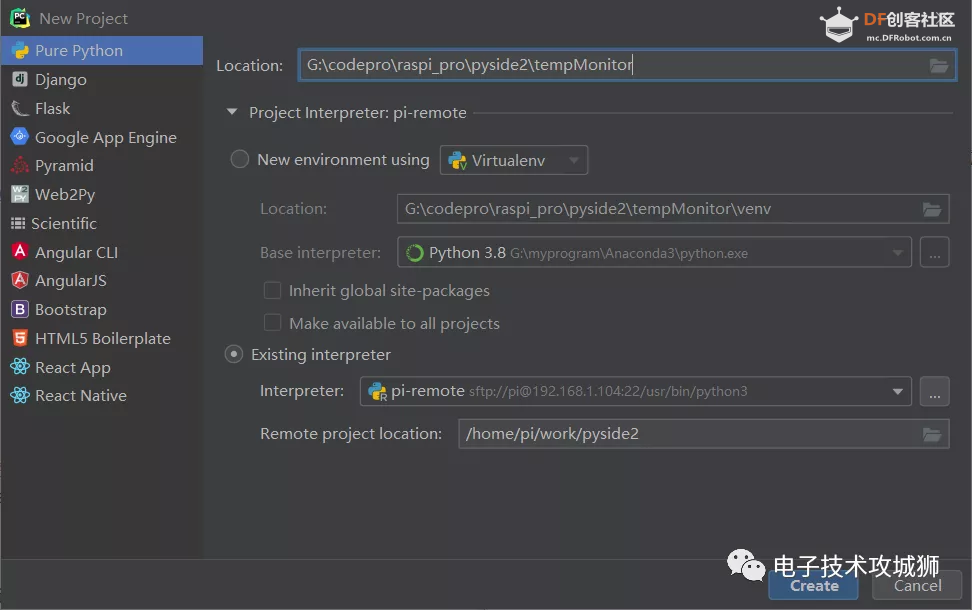
1.2、添加python主程序
tempMonitor.py 主程序如下:
- import math
- import os
- import sys
- import time
- from pathlib import Path
-
- from PySide2.QtCore import Qt, QObject, Slot
- from PySide2.QtQml import QQmlApplicationEngine
- from PySide2.QtWidgets import QApplication
-
- class Controler(QObject):
-
- def __init__(self):
- super().__init__()
- self.tempValue = 0
-
- @Slot()
- def exit(self):
- sys.exit()
-
- @Slot(result=float)
- def getTempValue(self):
- file_name = os.path.join("/", "mnt", "1wire", "uncached", "28.99E88D0D0000", "temperature")
-
- file_object = open(file_name, 'r')
- temp = file_object.read()
-
- self.tempValue = float(temp)
-
- print("tempvalue:",self.tempValue)
-
- file_object.close()
-
- return self.tempValue
-
- if __name__=='__main__':
-
- a = QApplication(sys.argv)
-
- a.setOverrideCursor(Qt.BlankCursor)
-
- engine = QQmlApplicationEngine()
-
- controler = Controler()
- context = engine.rootContext()
- context.setContextProperty("_Controler", controler)
-
- engine.load(os.fspath(Path(__file__).resolve().parent / "ui/monitor.qml"))
-
- if not engine.rootObjects():
- sys.exit(-1)
-
- sys.exit(a.exec_())
复制代码
- 在该程序中,建立一个 Controler 类,并实现了一个获取温度的方法,获取温度后就可以再qml界面中进行显示了。
1.3、添加界面文件- 在项目中添加ui文件夹,并新建main.qml文件; 参考代码如下:
复制代码
- 界面中使用了qml的一个组件 ChartView 用于显示温度的变化曲线;
- 使用qml的组件 Gauge 来显示变化刻度;
2、执行程序2.1、上传程序到树莓派
在工程上右键将这个项目文件上传到树莓派中:
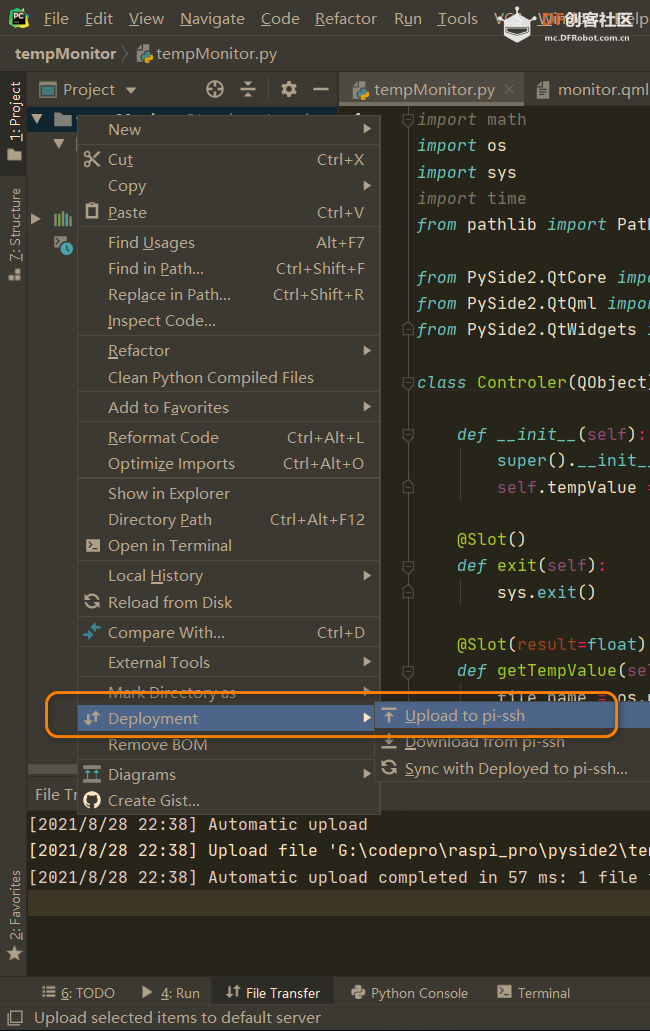
2.2、执行程序
上传后,在树莓派对应文件夹中,执行如下命令执行程序:
python3 tempMonitor.py
执行后可以看到显示如下:
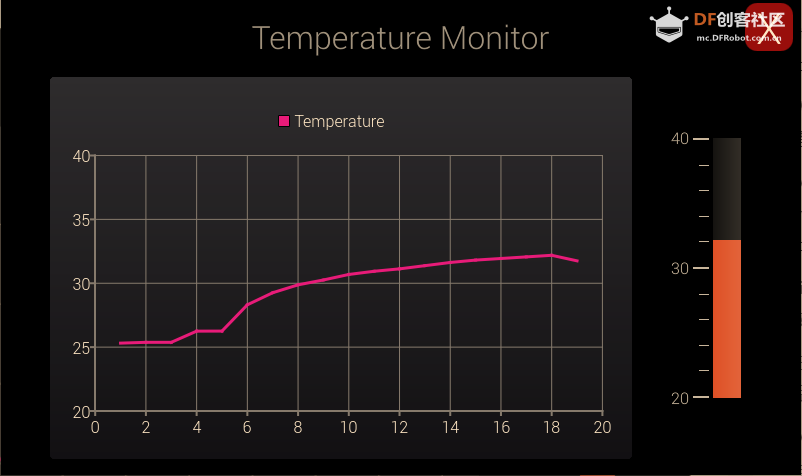
当用手接触DS18B20温度模块后,可以看到温度变化曲线:
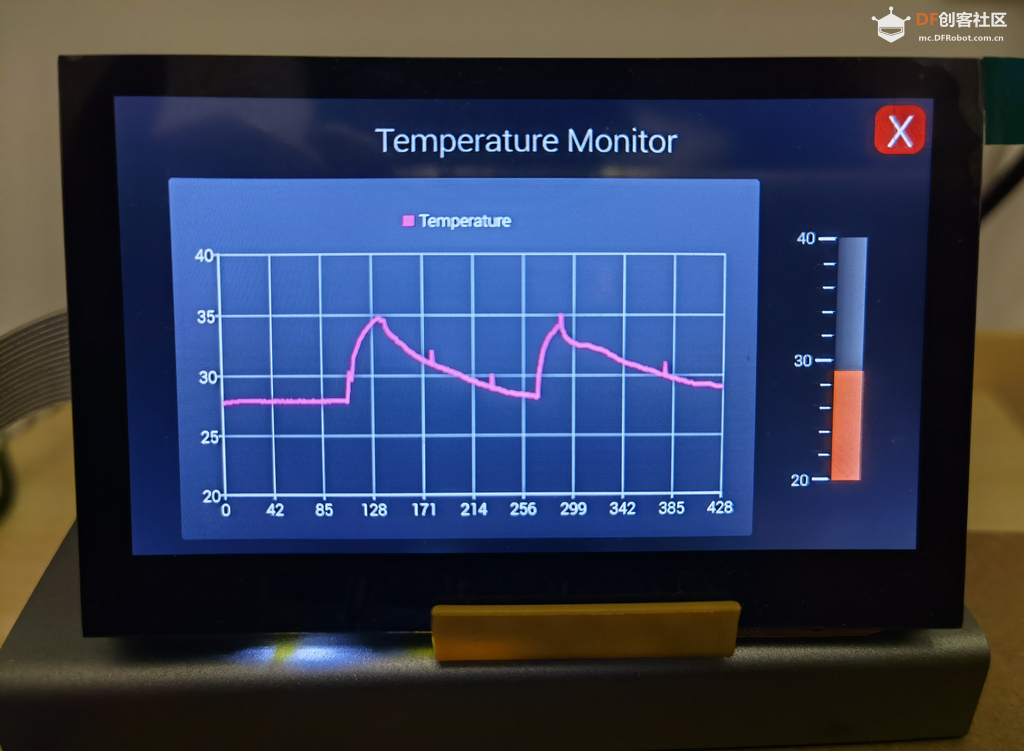
|