最近用ESP做大模型终端很流行,我们有个小群一些人也一直在摸索。
在实现大模型终端之前,准备先做对讲机。
今天这个教程就算半个对讲机吧。大家加个按钮控制,改成双向的应该就可以了。
声明:本教程代码由多个人使用多个大模型生成修改整合。
硬件:esp32一块,K10一块,麦克风模块INMP441一块,导线若干
编程环境:thonny,语言:micropython
固件:ESP32和ESP32S3标准固件(K10没用DF官方固件)
esp32接线图:
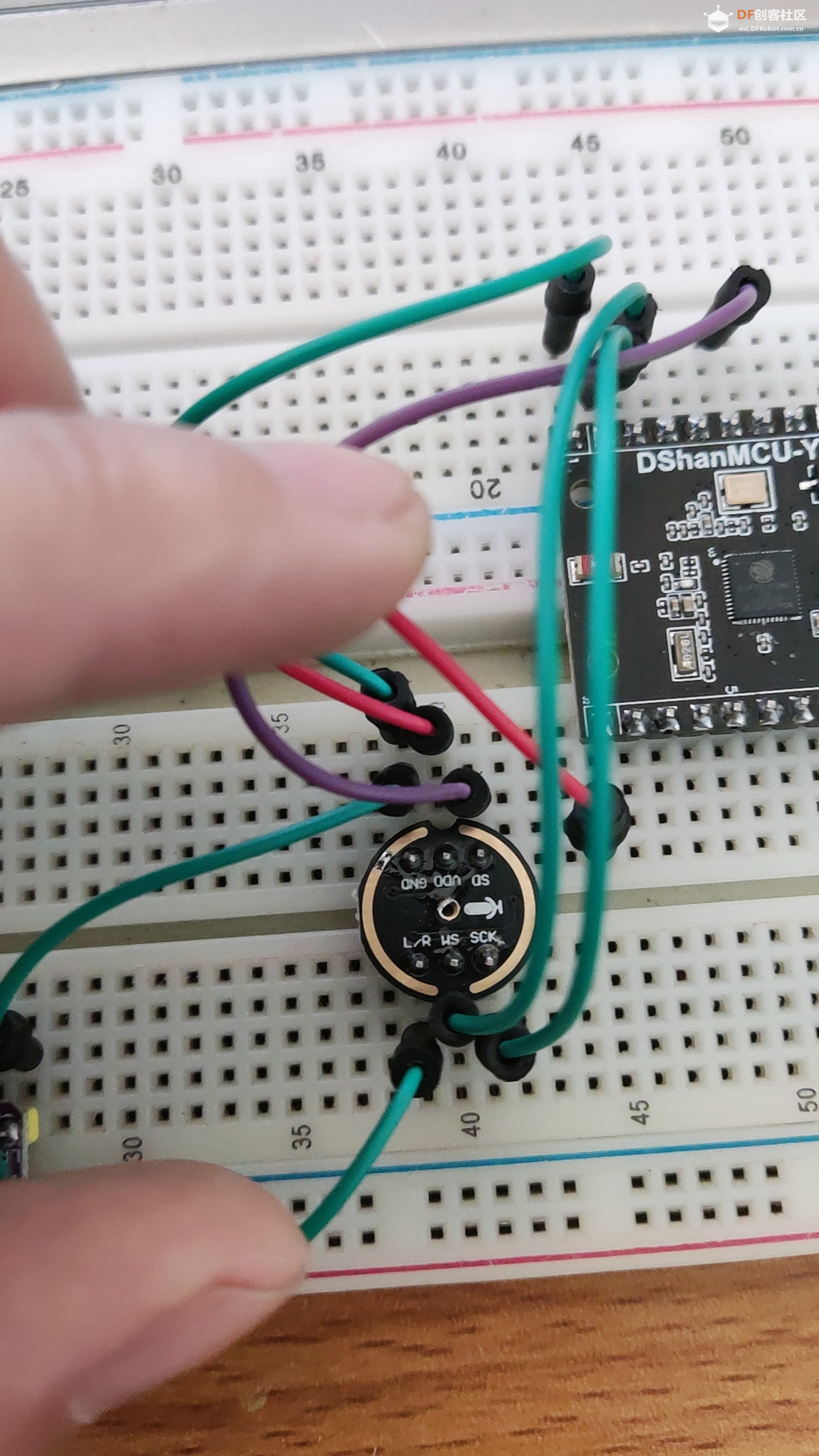 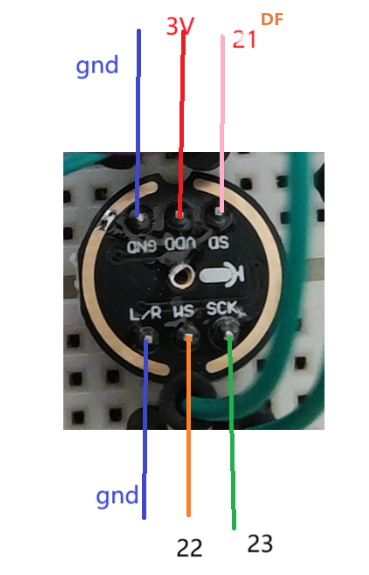
ESP32作为发射端,K10作为接收端(还不会用K10的麦克风)
发送端代码:
- from machine import I2S, Pin
- import network
- import socket
- import time
-
- # Network Configuration
- SSID = 'waoo2111280'
- PASSWORD = 'waoo2111280'
- SERVER_IP = '192.168.22.198'
- SERVER_PORT = 12345
-
- # I2S Configuration
- SCK_PIN = 23 # Serial clock output
- WS_PIN = 22 # Word clock output
- SD_PIN = 21 # Serial data output
-
- # Audio Configuration
- SAMPLE_RATE = 16000
- SAMPLE_BITS = 16 # Changed to 16-bit for better compatibility
- CHANNELS = 1 # Mono channel
- BUFFER_LENGTH = 2048 # Increased buffer size for better performance
-
- def connect_wifi():
- wlan = network.WLAN(network.STA_IF)
- wlan.active(True)
- if not wlan.isconnected():
- print('Connecting to WiFi...')
- wlan.connect(SSID, PASSWORD)
- while not wlan.isconnected():
- time.sleep(1)
- print('WiFi Connected')
- print('IP Address:', wlan.ifconfig()[0])
-
- def init_audio():
- audio_in = I2S(
- 0,
- sck=Pin(SCK_PIN),
- ws=Pin(WS_PIN),
- sd=Pin(SD_PIN),
- mode=I2S.RX,
- bits=SAMPLE_BITS,
- format=I2S.MONO, # Changed to mono for simplicity
- rate=SAMPLE_RATE,
- ibuf=BUFFER_LENGTH * 2 # Double buffer size
- )
- return audio_in
-
- def main():
- connect_wifi()
- audio = init_audio()
- sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
- audio_buf = bytearray(BUFFER_LENGTH)
-
- print('Starting audio capture and transmission...')
-
- try:
- while True:
- # Read audio data
- num_read = audio.readinto(audio_buf)
- if num_read > 0:
- # Send data in smaller chunks to prevent packet loss
- chunk_size = 1024
- for i in range(0, num_read, chunk_size):
- chunk = audio_buf[i:min(i + chunk_size, num_read)]
- sock.sendto(chunk, (SERVER_IP, SERVER_PORT))
- time.sleep_ms(1)
-
- except Exception as e:
- print('Error:', e)
- finally:
- audio.deinit()
- sock.close()
-
- if __name__ == '__main__':
- main()
-
复制代码
接收端代码:wifi_manager.py
- """WiFi connection management"""
- import network
- import time
-
- def connect_wifi(ssid, password):
- """Connect to WiFi network"""
- wlan = network.WLAN(network.STA_IF)
- wlan.active(True)
-
- if not wlan.isconnected():
- print('Connecting to WiFi...')
- wlan.connect(ssid, password)
- while not wlan.isconnected():
- time.sleep(1)
-
- print('Connected to WiFi')
- print('IP:', wlan.ifconfig()[0])
- return wlan
复制代码
audio_output.py- """Audio output handling using I2S and MAX98357A"""
- from machine import I2S, Pin
-
- class AudioOutput:
- def __init__(self, bclk_pin, ws_pin, data_pin, sample_rate, bits):
- """Initialize I2S output for MAX98357A"""
- self.audio_out = I2S(
- 1, # I2S ID (0 or 1)
- sck=Pin(bclk_pin), # Bit clock
- ws=Pin(ws_pin), # Word select
- sd=Pin(data_pin), # Data
- mode=I2S.TX, # Transmit mode
- bits=bits, # Sample size
- format=I2S.MONO, # Mono format
- rate=sample_rate, # Sample rate
- ibuf=10000 # Internal buffer size
- )
-
- def write(self, audio_data):
- """Write audio data to I2S DAC"""
- self.audio_out.write(audio_data)
-
- def deinit(self):
- """Clean up I2S resources"""
- self.audio_out.deinit()
复制代码
config.py
- """Configuration settings for the audio receiver"""
-
- # Network settings
- WIFI_SSID = 'waoo2111280'
- WIFI_PASSWORD = 'waoo2111280'
- UDP_PORT = 12345
- '''
- # I2S pins for MAX98357A
- BCLK_PIN = 26 # Bit clock
- WS_PIN = 25 # Word select / Left-right clock
- DATA_PIN = 22 # Data pin
- '''
-
- # 初始化引脚定义
- BCLK_PIN = 0 # 串行时钟输出
- WS_PIN = 38 # 字时钟
- DATA_PIN = 45 # 串行数据输出
-
- # Audio settings
- SAMPLE_RATE = 16000
- BITS_PER_SAMPLE = 16
- BUFFER_SIZE = 1024
复制代码
main.py
- """Main program for ESP32 audio receiver"""
- import socket
- from config import *
- from wifi_manager import connect_wifi
- from audio_output import AudioOutput
-
- def main():
- # Connect to WiFi
- connect_wifi(WIFI_SSID, WIFI_PASSWORD)
-
- # Initialize audio output
- audio = AudioOutput(
- bclk_pin=BCLK_PIN,
- ws_pin=WS_PIN,
- data_pin=DATA_PIN,
- sample_rate=SAMPLE_RATE,
- bits=BITS_PER_SAMPLE
- )
-
- # Setup UDP socket
- sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
- sock.bind(('0.0.0.0', UDP_PORT))
- print(f'Listening on port {UDP_PORT}')
-
- try:
- while True:
- try:
- # Receive audio data
- data, addr = sock.recvfrom(BUFFER_SIZE)
- # Play audio through MAX98357A
- audio.write(data)
- except Exception as e:
- print('Error:', e)
-
- finally:
- audio.deinit()
- sock.close()
-
- if __name__ == '__main__':
- main()
-
复制代码
修改wifi账号密码,把两端各自的代码上传到板子上,运行,先运行接收端,获得IP地址填入发送端代码。
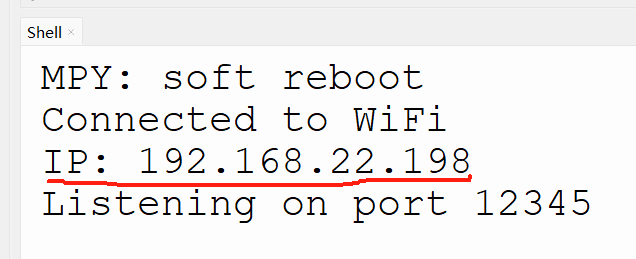
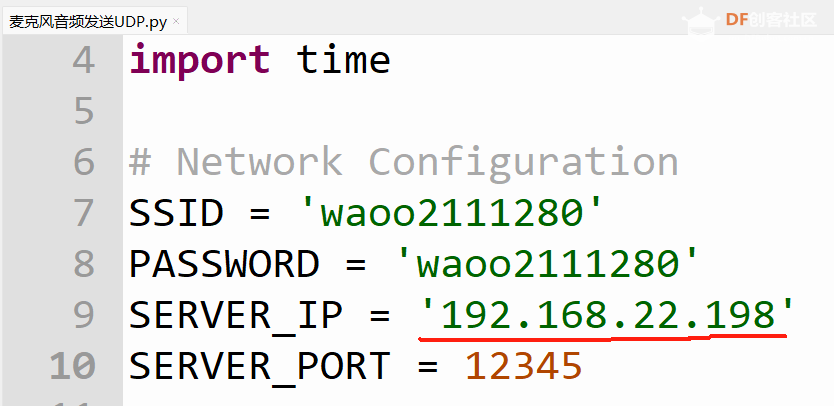
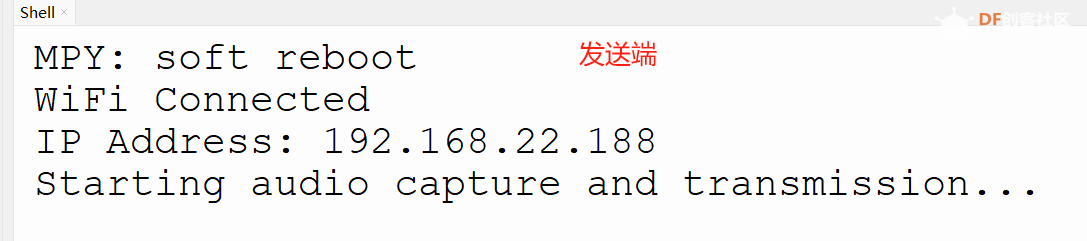
视频
|