本帖最后由 yiD31Agzr1kQ 于 2025-5-3 05:39 编辑
树莓派 Beetle-RP2350 MacOS开发环境搭建及跑分测试
近期有幸体验了DFRobot推出的Beetle-RP2350微型开发板,这款仅硬币大小的开发板给我留下了深刻印象。作为一款基于树莓派RP2350微控制器的产品,它拥有以下核心特性:
- 双核双架构,Arm Cortex-M33或Hazard3 RISC-V内核
- 150MHz主频,520KB RAM,2MB flash。
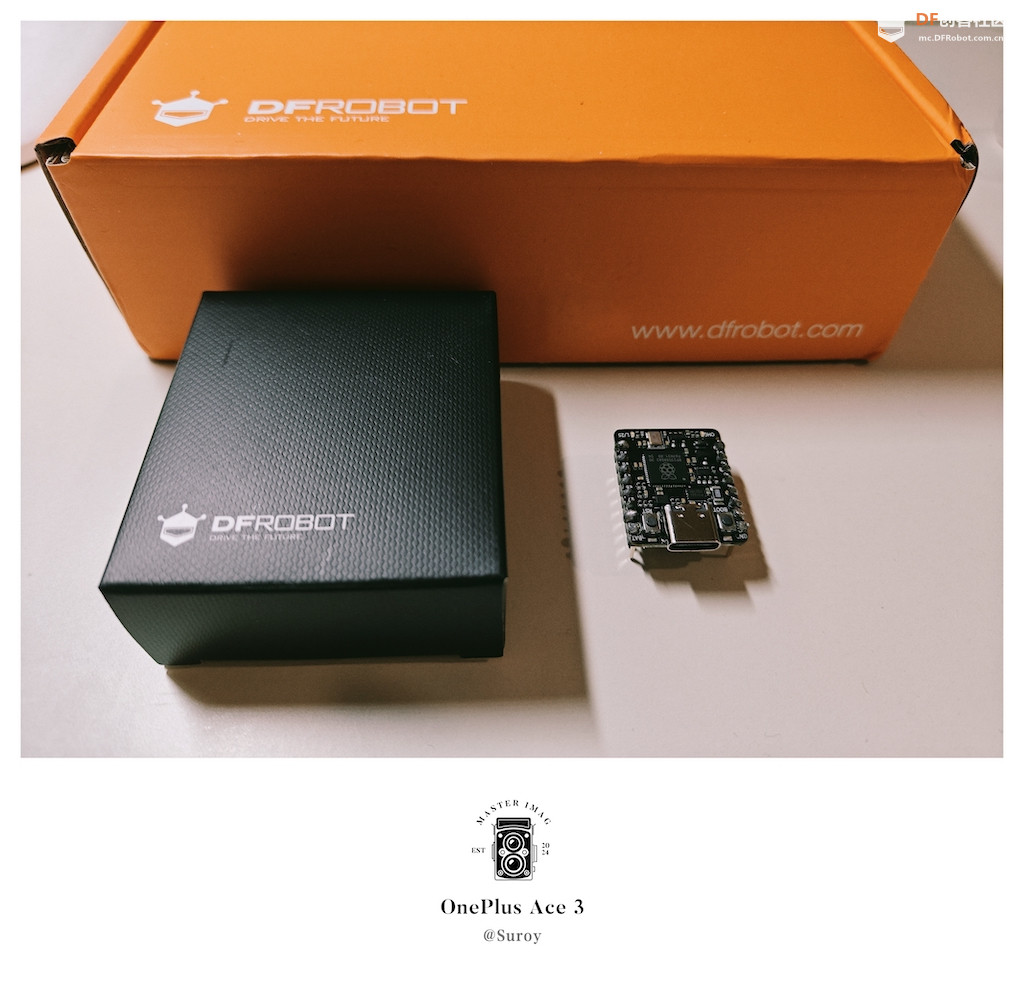
浏览了一下社区中关于Arduino生态的内容较为丰富,但出于对MicroPython的偏好,我决定采用MicroPython作为开发环境。本文将介绍在MacOS系统下搭建开发环境的完整过程,并通过基准测试其性能表现。
前期准备
开发环境如下
- 系统: MacOS Catalina 10.15.7
- IDE: PyCharm Pro + MicroPython + Tabby(终端工具)
- 硬件: Beetle-RP2350
相关资料
1. 官方资料: https://www.raspberrypi.com/documentation/microcontrollers/silicon.html#rp2350
2. 固件地址:
- https://micropython.org/download/?mcu=rp2350
- https://micropython.org/download/RPI_PICO2/
3. MicroPython 教程地址: https://docs.micropython.org/en/latest/rp2/quickref.html
环境搭建
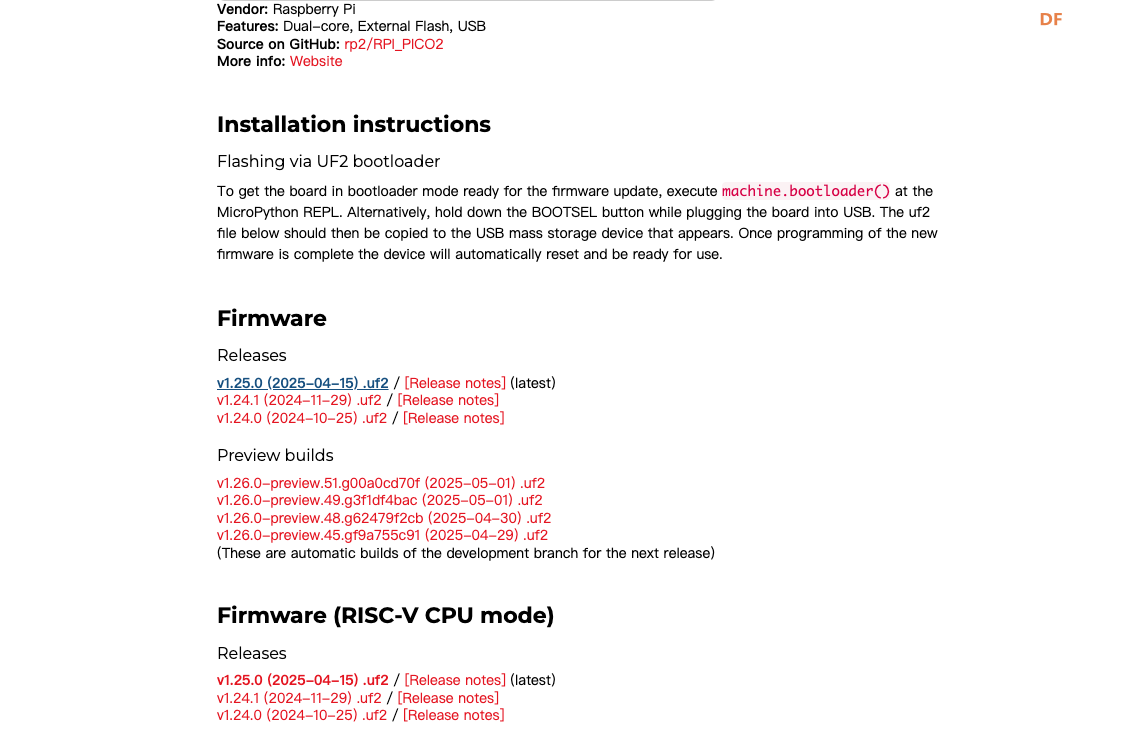
根据 MicroPython 官方文档指导,可以将RP2350连上USB进入大容量设备模式(类似于U盘),将下载好的固件( RPI_PICO2-20250415-v1.25.0.uf2 )拷贝进去即烧录成功自动重启。
1. 进入大容量模式:按住 BOOT 不放 + 点按 RST
2. 拖动拷贝固件到 `RP2350` 盘符,完成之后会自动弹出该盘符
3. 按下 RST 复位
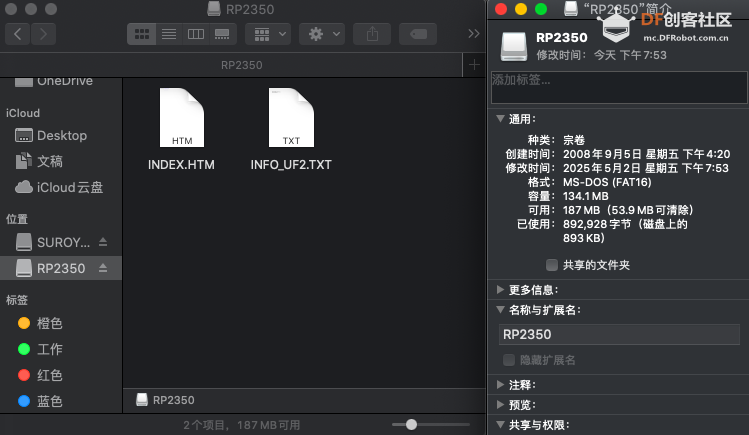
上机测试
点灯测试
-
- from machine import Pin
- import time
-
- LED = Pin(25, Pin.OUT)
-
- while True:
- LED.on()
- time.sleep(1)
- LED.off()
- time.sleep(1)
复制代码
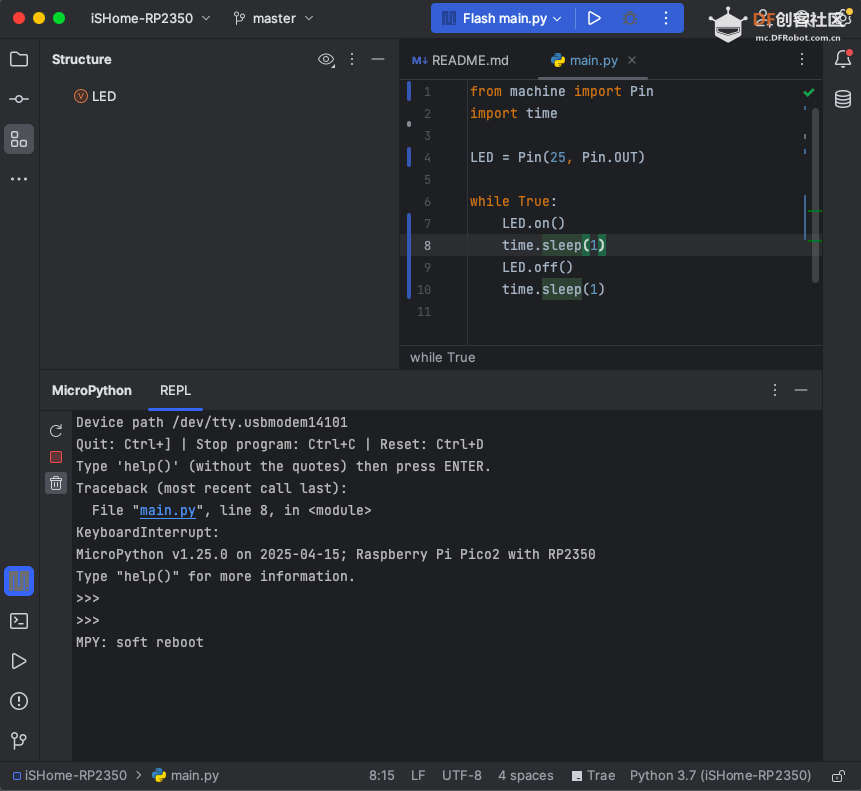
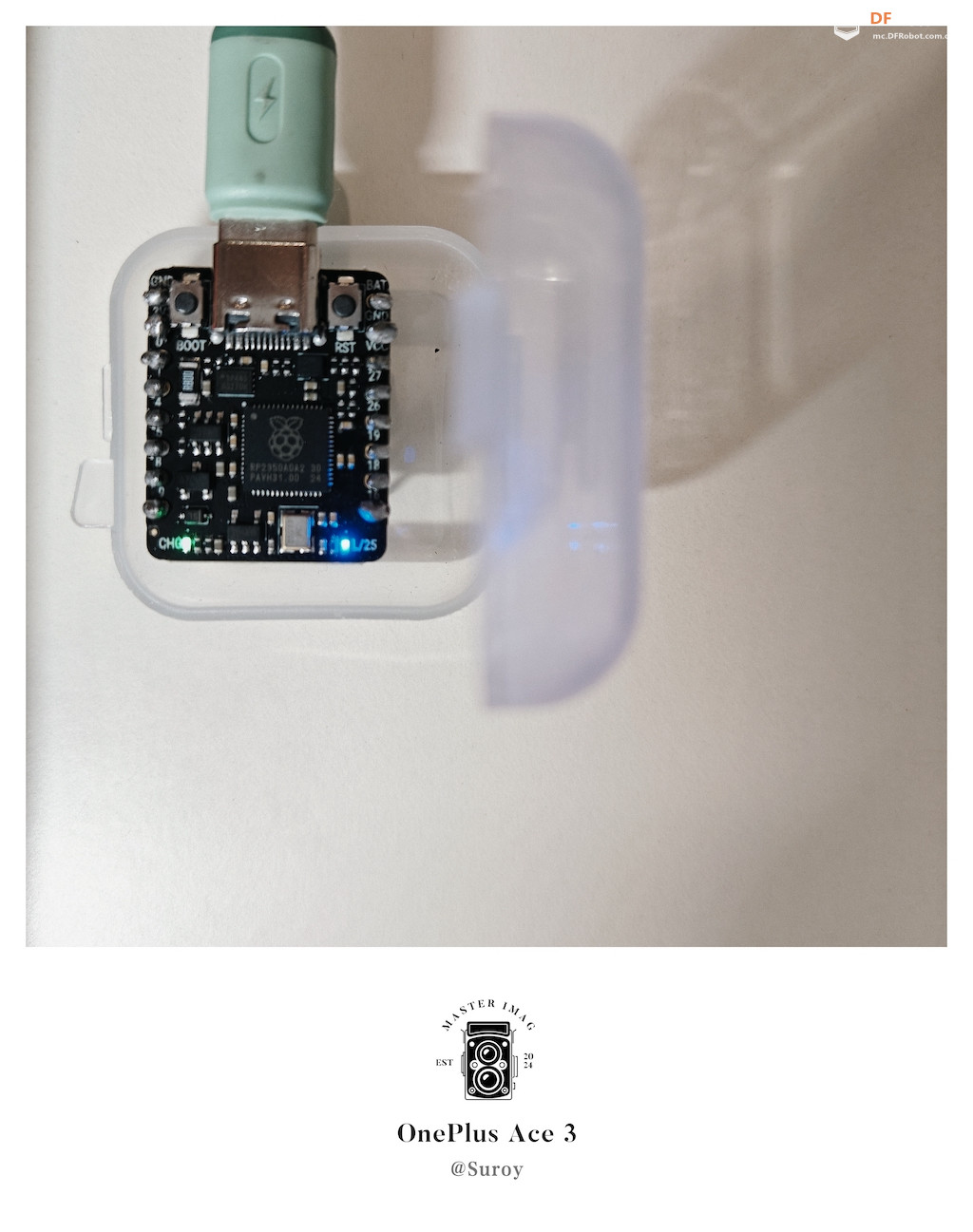
跑分测试
摘录了以下RP2350和RP2040的性能表格,但是实际我这边测试出来与给出有点出入,仅供参考,建议以C/C++进行基准测试。
测试涵盖:
1. 基础运算性能(整数/浮点)
2. 复杂算法处理(Mandelbrot集计算)
3. 高精度计算(π值计算)
+ BenchMark: https://github.com/shaoziyang/micropython_benchmarks

注: 时间单位ms, 越小则性能越好。
BenchMark:
-
- '''
- Simple benchmark for micropython and circuitpython.
- Compare the performance of different MCUs by performing operations such as addition, multiplication, exponentiation, complex numbers, and pi.
- '''
- import sys
- import gc
-
- try:
- from time import ticks_ms, ticks_diff
- import machine
-
- TEST_ENV = 'micropython'
-
-
- def freq():
- try:
- f = machine.freq() // 1000000
- except:
- f = machine.freq()[0] // 1000000
- return f
- except:
- from time import monotonic_ns
-
-
- def ticks_ms():
- return monotonic_ns() // 1000000
-
-
- def ticks_diff(T2, T1):
- return T2 - T1
-
-
- try:
- import board
-
- TEST_ENV = 'circuitpython'
-
- import microcontroller
-
-
- def freq():
- return microcontroller.cpu.frequency // 1000000
-
- except:
- TEST_ENV = 'OTHER'
-
- try:
- import psutil
-
-
- def freq():
- return psutil.cpu_freq().max
- except:
- def freq():
- return 'unknow'
-
-
- def memory():
- try:
- r = gc.mem_free() + gc.mem_alloc()
- except:
- try:
- r = psutil.virtual_memory().total
- except:
- r = 'unkonw'
- return r
-
-
- PLATFORM = sys.platform
- VERSION = sys.version
- FREQ = freq()
- MEMORY = memory()
-
-
- def run_test(func, *param):
- gc.collect()
- try:
- t1 = ticks_ms()
- if param == None:
- func()
- else:
- func(*param)
- t2 = ticks_ms()
- dt = ticks_diff(t2, t1)
- print('Calculation time:', dt, 'ms\n')
- return dt
- except:
- print('Error occurred during operation!')
-
-
- def mandelbrot_test(p):
- iter = p[0]
-
- def in_set(c):
- z = 0
- for i in range(iter):
- z = z * z + c
- if abs(z) > 4:
- return False
- return True
- r = ''
- for v in range(31):
- for u in range(81):
- if in_set((u / 30 - 2) + (v / 15 - 1) * 1j):
- r += ' '
- else:
- r += '#'
- r += '\n'
- if len(p) > 1 and p[1]:
- print(r)
-
-
- def pi_test(p):
- iter = p[0]
- extra = 8
- one = 10 ** (iter + extra)
- t, c, n, na, d, da = 3 * one, 3 * one, 1, 0, 0, 24
-
- while t > 1:
- n, na, d, da = n + na, na + 8, d + da, da + 32
- t = t * n // d
- c += t
- return c // (10 ** extra)
-
-
- def add_test(p):
- for i in range(p[0]):
- C = p[1] + p[2]
-
-
- def mul_test(p):
- for i in range(p[0]):
- C = p[1] * p[2]
-
-
- def div_test(p):
- for i in range(p[0]):
- C = p[1] / p[2]
-
-
- def pow_test(p):
- for i in range(p[0]):
- C = p[1] ** p[2]
-
-
- INT_ADD_TEST_LIST = ('Integer addition {} times', add_test, [12345678, 56781234], 10000, 100000, 1000000)
- INT_MUL_TEST_LIST = ('Integer multiplication {} times', mul_test, [12345678, 56781234], 10000, 100000, 1000000)
- INT_DIV_TEST_LIST = ('Integer division {} times', div_test, [99999991, 45481], 10000, 100000, 1000000)
- FLOAT_ADD_TEST_LIST = ('Float addition {} times', add_test, [12345678.1234, 56781234.5678], 10000, 100000, 1000000)
- FLOAT_MUL_TEST_LIST = (
- 'Float multiplication {} times', mul_test, [12345678.1234, 56781234.5678], 10000, 100000, 1000000)
- FLOAT_DIV_TEST_LIST = ('Float division {} times', div_test, [99999991.2345, 45481.1357], 10000, 100000, 1000000)
- POWER_TEST_LIST = ('Power calculation {} times', pow_test, [1234.5678, 2.3456], 10000, 100000, 1000000)
- MAND_TEST_LIST = ('Mandelbrot iterating {} times', mandelbrot_test, [], 100, 500, 5000)
- PI_TEST_LIST = ('Pi Calculation {} bits', pi_test, [], 1000, 5000, 10000, 100000, 200000)
-
- TEST_LIST = (
- INT_ADD_TEST_LIST, INT_MUL_TEST_LIST, INT_DIV_TEST_LIST,
- FLOAT_ADD_TEST_LIST, FLOAT_MUL_TEST_LIST, FLOAT_DIV_TEST_LIST,
- POWER_TEST_LIST,
- MAND_TEST_LIST,
- PI_TEST_LIST
- )
-
- tr = []
-
-
- def run():
- global tr
-
- for TEST in TEST_LIST:
- for item in TEST:
- if type(item) == int:
- print(TEST[0].format(item))
- p = TEST[2].copy()
- p.insert(0, item)
- r = run_test(TEST[1], p)
- tr.append([TEST[0].format(item), str(r)])
-
-
- def print_result():
- r1 = '| item | Platform | Version | Frequency | Memory |'
- r2 = '| - | - | - | - | - |'
- r3 = '| {} | {} | {} | {} | {} |'.format('result', PLATFORM, VERSION, FREQ, MEMORY)
-
- for i in range(len(tr)):
- r1 += ' {} |'.format(tr[0])
- r2 += ' - |'
- r3 += ' {} |'.format(tr[1])
-
- print(r1)
- print(r2)
- print(r3)
-
-
- ###############################################################################
-
- print('\n\n')
- print('##############')
- print('# Begin test #')
- print('##############')
-
- print('\nEnvironment:', TEST_ENV)
- print('Platform:', PLATFORM)
- print('Version:', VERSION)
- print('Frequency:', FREQ)
- print('Memory:', MEMORY)
- print()
-
- run()
-
- print('\nTest finished.')
- print('==============')
-
- print_result()
复制代码
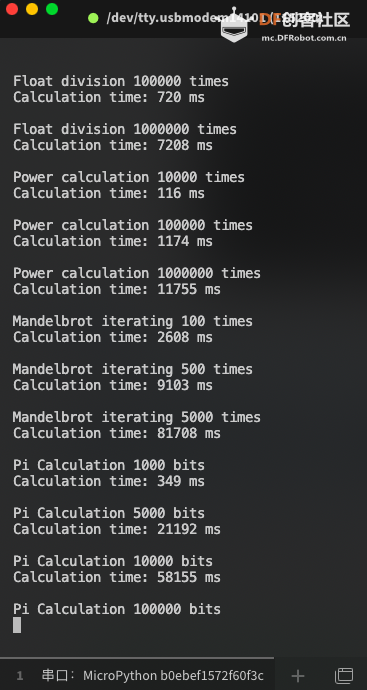
串口运行结果日志:
-
- Calculation time: 4259 ms
-
- Integer multiplication 10000 times
-
- Calculation time: 161 ms
-
- Integer multiplication 100000 times
-
- Calculation time: 1628 ms
-
- Integer multiplication 1000000 times
-
- Calculation time: 16284 ms
-
- Integer division 10000 times
-
- Calculation time: 62 ms
-
- Integer division 100000 times
-
- Calculation time: 639 ms
-
- Integer division 1000000 times
-
- Calculation time: 6400 ms
-
- Float addition 10000 times
-
- Calculation time: 69 ms
-
- Float addition 100000 times
-
- Calculation time: 708 ms
-
- Float addition 1000000 times
-
- Calculation time: 7094 ms
-
- Float multiplication 10000 times
-
- Calculation time: 70 ms
-
- Float multiplication 100000 times
-
- Calculation time: 710 ms
-
- Float multiplication 1000000 times
-
- Calculation time: 7108 ms
-
- Float division 10000 times
-
- Calculation time: 71 ms
-
- Float division 100000 times
-
- Calculation time: 720 ms
-
- Float division 1000000 times
-
- Calculation time: 7208 ms
-
- Power calculation 10000 times
-
- Calculation time: 116 ms
-
- Power calculation 100000 times
-
- Calculation time: 1174 ms
-
- Power calculation 1000000 times
-
- Calculation time: 11755 ms
-
- Mandelbrot iterating 100 times
-
- Calculation time: 2608 ms
-
- Mandelbrot iterating 500 times
-
- Calculation time: 9103 ms
-
- Mandelbrot iterating 5000 times
-
- Calculation time: 81708 ms
-
- Pi Calculation 1000 bits
-
- Calculation time: 349 ms
-
- Pi Calculation 5000 bits
-
- Calculation time: 21192 ms
-
- Pi Calculation 10000 bits
-
- Calculation time: 58155 ms
-
- Pi Calculation 100000 bits
复制代码
跑分结论大致如下:
- CircuitPython(适用于大多数场景): 浮点运算速度快、内存占用低,运行复杂算法(如分形、高精度计算),推荐科学计算、图形渲染等场景。
- MicroPython: 整数运算速度快(如嵌入式控制、传感器数据处理),短任务响应更敏感(如快速初始化)。
尾言
通过本次完整的开发体验,Beetle-RP2350展现出了优异的性能表现和开发便利性。其双核架构在保持低功耗的同时,提供了显著的性能提升。MicroPython生态的成熟度也使得快速原型开发成为可能。对于嵌入式开发者而言,这款开发板在物联网终端、智能控制等领域都具有广阔的应用前景。
> 后续计划:将进行环境监测系统实验,欢迎关注项目更新。
作者: Suroy |个人博客同步更新: https://suroy.cn/embeded/raspberry-pi-beetlerp2350-macos-development-environment-setup-and-benchmark-testing.html
|