本帖最后由 红明 于 2014-1-25 22:44 编辑
Arduino和Leap motion连接在同一台电脑上,通过Processin将手的位置传回,驱动Servo转动。
更新 2014-01-25
图中的LED Matrix替换为一个单色二极管。下面的图和代码也是依此更改。
原理:
Leap Motion捕捉手的位置,通过Processing取得部分数据,并传输给Arduino以控制两个舵机和一个LED。
主要硬件:
一个Arduino UNO
两个舵机Servo
一个红LED
一个200欧姆电Resistor
一个面包板
一包连接线
一个Leap Motion
与软硬件有关的网址
Leap Motion Dev社区
https://developer.leapmotion.com/
Processing
http://www.processing.org
LeapMotionForProcessing by Darius Morawiec
https://github.com/voidplus/leap-motion-processing
Arduino Servo
http://arduino.cc/en/reference/servo
连线图
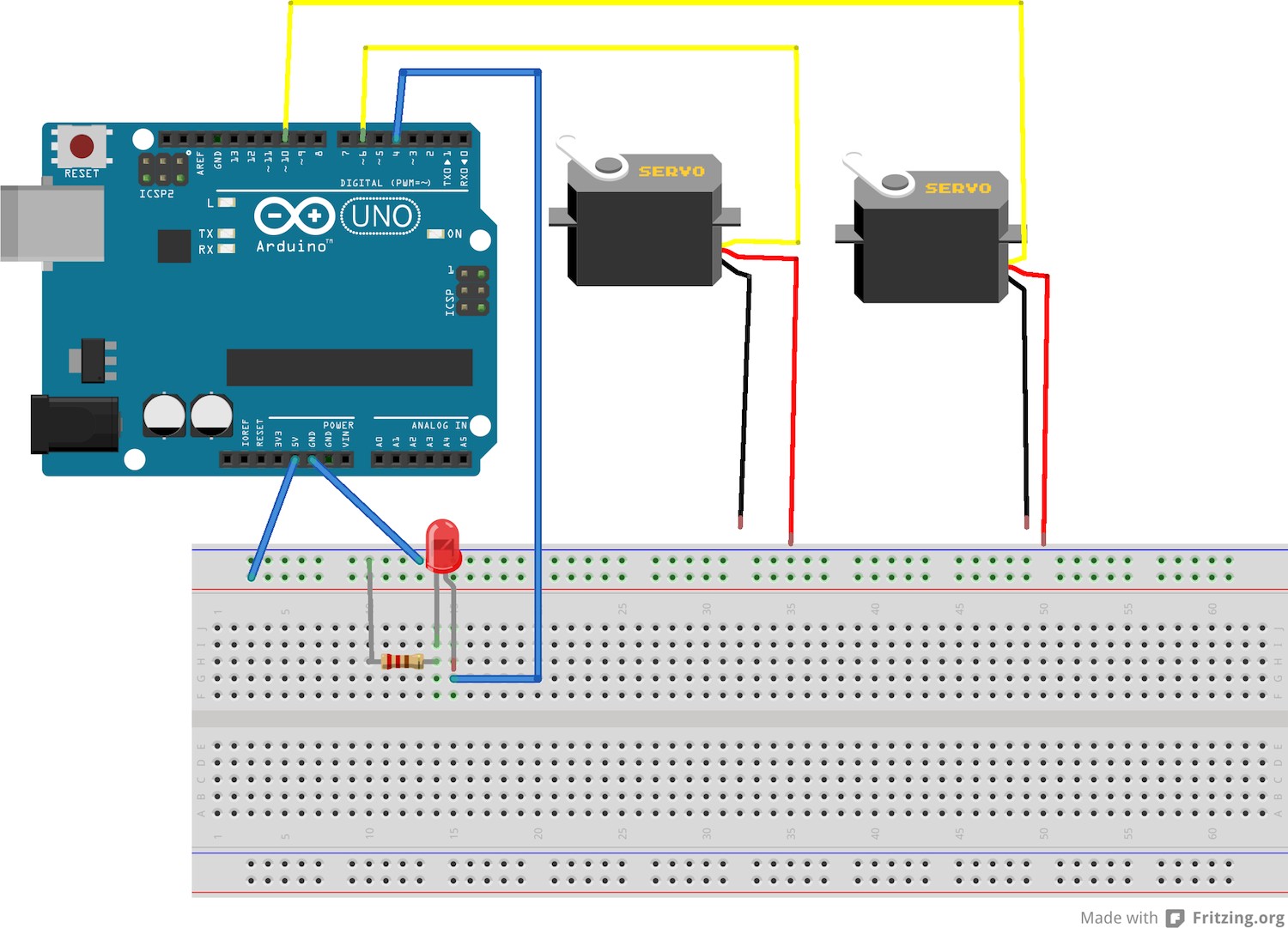
Arduino代码
- #include <Servo.h>
-
- Servo leapservo;
- Servo leapservo2;
- int servopin=6;
- int servo2pin=10;
- int breadboardledpin = 4;
-
- void setup(){
-
- Serial.begin(9600);
- leapservo2.attach(servo2pin);
- leapservo.attach(servopin);
- pinMode(breadboardledpin,OUTPUT);
- }
-
- void loop(){
- if(Serial.available()){
- char buffer[3];
- Serial.readBytes(buffer,3);
-
- int leaphandx = buffer[0];
- if (leaphandx<=-76 && leaphandx >-128){
- leaphandx = 256-abs(leaphandx);
- }
- else if (leaphandx > -76 && leaphandx<0){
- leaphandx = 179;
- }
- else{
- leaphandx = buffer[0];
- }
-
- int leaphandy = buffer[1];
- if (leaphandy<=-76 && leaphandy >-128){
- leaphandy = 256-abs(buffer[0]);
- }
- else if (leaphandy > -70 && leaphandy<0){
- leaphandy = 179;
- }
- else{
- leaphandy = buffer[1];
- }
-
- int breadboardled = buffer[2];
- if(breadboardled>=0 && breadboardled<110){
- digitalWrite(breadboardledpin,HIGH);
- }
- else{
- digitalWrite(breadboardledpin,LOW);
- }
-
- leapservo2.write(leaphandx);
- leapservo.write(leaphandy);
-
- }
- Serial.flush();
- }
复制代码
Processing代码
- import processing.serial.*;
- import de.voidplus.leapmotion.*;
- import development.*;
- Serial port;
-
- LeapMotion leap;
- float processhandx;
- float processhandy;
- float processhandz;
-
- void setup() {
- size(800, 500, P3D);
- background(255);
- noStroke();
- fill(50);
- port = new Serial(this, Serial.list()[2], 9600);
- leap = new LeapMotion(this);
- }
-
- void draw() {
- background(100);
- int fps = leap.getFrameRate();
-
- for (Hand hand : leap.getHands()) {
- hand.draw();
- PVector hand_position = hand.getPosition();
- PVector hand_dynamics = hand.getDynamics() ;
- int finger_count = hand.countFingers();
- boolean get_finger = hand.hasFingers();
- if (hand_position.x<20) {
- processhandx = 0;
- }
- else if (hand_position.x>=780) {
- processhandx = 179;
- }
- else {
- processhandx = map(hand_position.x, 20, 780, 0, 179);
- }
-
- if (hand_position.y<50) {
- processhandy = 0;
- }
- else if (hand_position.y>=450) {
- processhandy = 179;
- }
- else {
- processhandy = map(hand_position.y, 50, 450, 0, 179);
- }
-
- if (hand_position.z< -50) {
- processhandz = 0;
- }
- else if (hand_position.z>=50) {
- processhandz = 179;
- }
- else {
- processhandz = map(hand_position.z, -50, 50, 0, 179);
- }
-
- byte[] q = {
- byte(processhandx), byte(processhandy), byte(processhandz)
- };
- println(q);
-
- port.write(q);
- }
- }
复制代码
|