本帖最后由 麦壳maikemaker 于 2024-4-9 21:28 编辑
幸运的获得了试用名额,正好最近在上micropython+APP inventor的课程,所以做一个APP通过MQTT远程控制彩灯的小实验。
硬件:FireBeetle 2 ESP32 C6,WS2812灯环
程序语言:micropython
APP开发环境:APP inventor2
电路图:
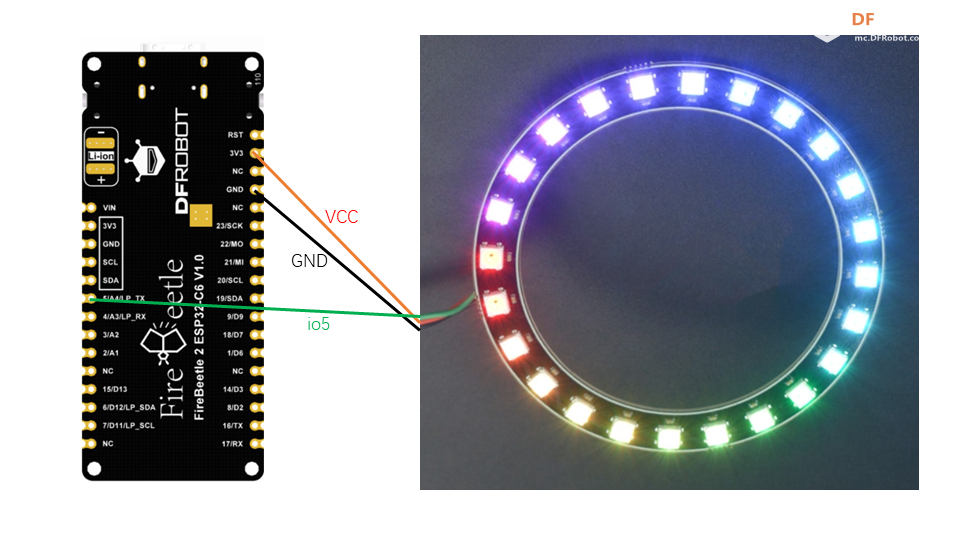
C6代码:
- '''
- 实验名称:自建APP控制RGB灯
- 20240409
- '''
- import network,time
- from umqtt.simple import MQTTClient #导入MQTT板块
- from machine import Pin,Timer
- import neopixel #导入ws2812彩灯库
- import urandom #导入随机数库
-
-
- RGBIO = Pin(5) # 彩灯控制引脚
- LED_NUM = 12 # 彩灯的数量
-
- LED = neopixel.NeoPixel(pin=RGBIO, n=LED_NUM, timing=1) # 创建控制对象
-
- LED.fill((0, 0, 0)) # GRB填充数据(RGB顺序, 0为不亮,255为全亮)
- LED.write() # 写入数据(生效)
-
-
- #WIFI连接函数
- def WIFI_Connect():
-
- WIFI_LED=Pin(15, Pin.OUT) #初始化WIFI指示灯
-
- wlan = network.WLAN(network.STA_IF) #STA模式
- wlan.active(True) #激活接口
- start_time=time.time() #记录时间做超时判断
-
- if not wlan.isconnected():
- print('connecting to network...')
- wlan.connect('waoo2111280', 'waoo2111280') #输入WIFI账号密码
-
- while not wlan.isconnected():
-
- #LED闪烁提示
- WIFI_LED.value(1)
- time.sleep_ms(300)
- WIFI_LED.value(0)
- time.sleep_ms(300)
-
- #超时判断,15秒没连接成功判定为超时
- if time.time()-start_time > 15 :
- print('WIFI Connected Timeout!')
- break
-
- if wlan.isconnected():
- #LED点亮
- WIFI_LED.value(1)
- #串口打印信息
- print('network information:', wlan.ifconfig())
- LED.fill((0, 255, 0)) #联网成功显示绿色
- LED.write()
- return True
-
- else:
- LED.fill((255, 0, 0))#联网失败显示红色
- LED.write()
- return False
- #控制全部灯珠
- def allled(msg):
- #{200,200,200}数据结构RGB
- print(msg[3 : (len(msg) - 2)])
- RGB = msg[3 : (len(msg) - 2)].split(',')
- LED.fill((int(RGB[0]), int(RGB[1]), int(RGB[2])))
- LED.write()
- #控制单个灯珠
- def oneled(msg):
- #{200,200,200,11}数据结构RGB,灯珠位置
- print(msg[3 : (len(msg) - 2)])
- RGB = msg[3 : (len(msg) - 2)].split(',')
- LED[int(RGB[3])]=((int(RGB[0]), int(RGB[1]), int(RGB[2])))
- LED.write()
-
- #设置MQTT回调函数,有信息时候执行
- def MQTT_callback(topic, msg):
- print('topic: {}'.format(topic))
- print('msg:{}'.format(msg))
- #根据TOPIC调用相应函数,并传入msg
- if "b'dfrobot/fire/beetle/all'"== '{}'.format(topic):
- allled('{}'.format(msg))
- elif "b'dfrobot/fire/beetle/one'"== '{}'.format(topic):
- oneled('{}'.format(msg))
-
- #接收数据任务
- #300MS检查一次消息
- def MQTT_Rev(tim):
- client.check_msg()
-
-
- #执行WIFI连接函数并判断是否已经连接成功
- if WIFI_Connect():
-
- SERVER = 'broker.emqx.io'#MQTT服务器
- PORT = 1883 #端口
- CLIENT_ID = '8b7562984asdfg' # 客户端ID
- #全部灯
- TOPIC = 'dfrobot/fire/beetle/all' # TOPIC名称
- #单个灯
- TOPIC1 = 'dfrobot/fire/beetle/one' # TOPIC名称
-
- client = MQTTClient(CLIENT_ID, SERVER, PORT) #建立客户端对象
- client.set_callback(MQTT_callback) #配置回调函数
- client.connect()#连接服务器
- print('connect')
- client.subscribe(TOPIC) #订阅主题
- client.subscribe(TOPIC1) #订阅主题
- print('subscribe')
- #开启RTOS定时器,编号为-1,周期300ms,执行socket通信接收任务
- #C6定时器编号0
- tim = Timer(0) #创建定时器
- tim.init(period=300, mode=Timer.PERIODIC,callback=MQTT_Rev)
-
复制代码
APP界面设计:
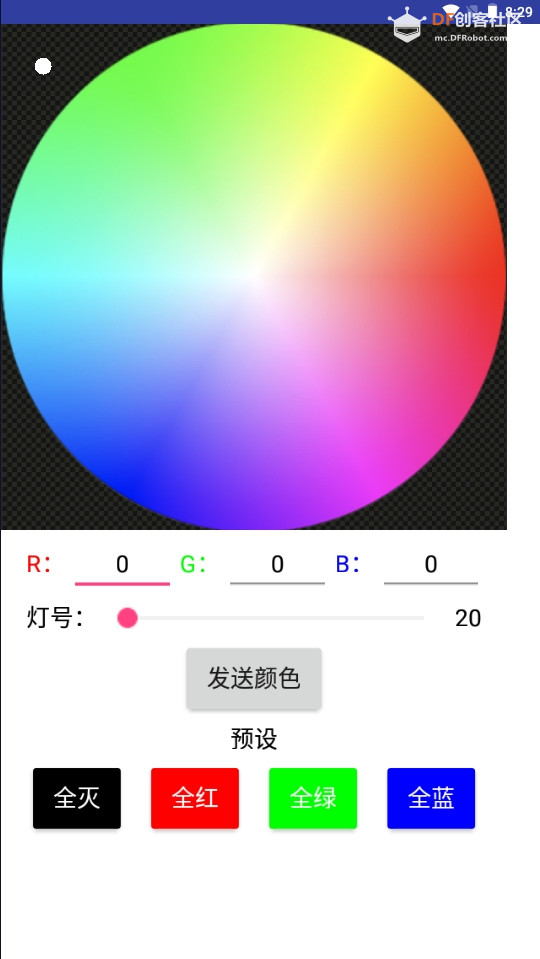
APP程序设计:
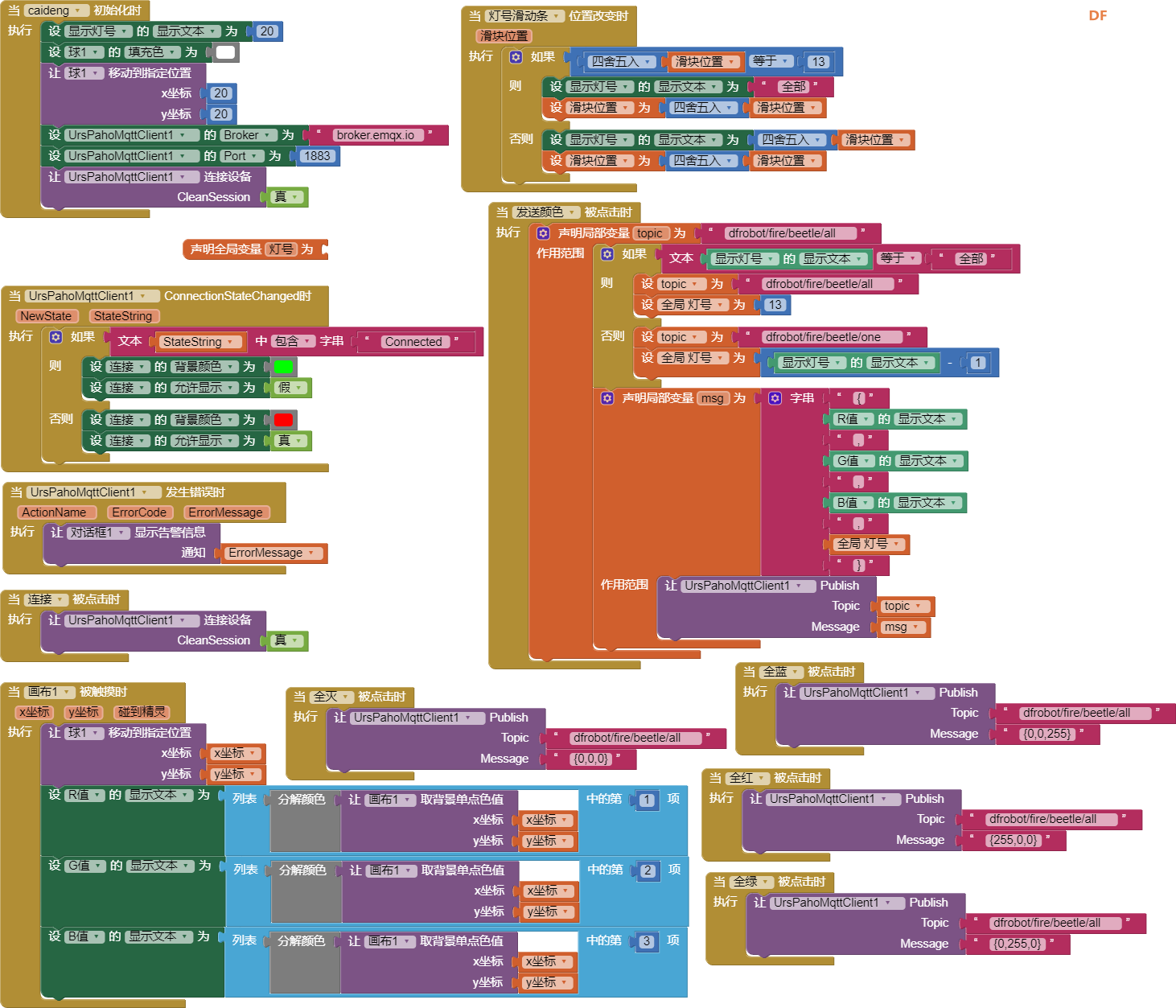
效果:
附:
FireBeetle 2 ESP32 C6: https://www.dfrobot.com.cn/goods-3825.html
彩灯: https://www.dfrobot.com.cn/goods-3472.html
固件:https://mc.dfrobot.com.cn/thread-318231-1-1.html
APPinventor2:http://ai2.17coding.net/?locale=zh_CN
|