本帖最后由 云天 于 2021-8-16 13:07 编辑
【晃动灯】
1、数字震动传感器
本开关在静止时为开路(OFF)状态,当受到外力碰触而达到适当震动力时,或移动速度达到适当离(偏)心力时,导电接脚会发生瞬间导通(ON)状态,使电气特性改变,而当外力消失时电气特性恢复开路(OFF)状态。无方向性,任何角度均可以触发工作。
-
- #include <Adafruit_NeoPixel.h>
-
- #define PIN_LED 16
- #define NUM_LED 1
- int shake =25;
- Adafruit_NeoPixel pixels(NUM_LED, PIN_LED, NEO_GRB + NEO_KHZ800);
- void setup() {
- // put your setup code here, to run once:
- pixels.begin();
- pinMode(shake, INPUT);
- pinMode(16, OUTPUT);
- Serial.begin(9600);
-
- }
-
- void loop() {
- pixels.clear();
- int State = digitalRead(shake);
- Serial.println(State);
- if(State==0){
- pixels.setBrightness(200);
- pixels.setPixelColor(0, pixels.Color(int(random(0,255)), int(random(0,255)),int( random(0,255))));
- pixels.show();
- delay(2000);
- pixels.setBrightness(0);
- pixels.show();
-
- }
- }
复制代码
LIS2DW12三轴加速度计是一款超低功耗的线性加速度计,该传感器拥有两个独立的可编程中断及专用内部引擎,可实现超多功能,例如自由落体检测、纵向/横向检测、朝向检测、可配置的单击/双击识别、运动检测、运动唤醒以实现高级省电等,我们为您提供了以上功能的示例程序,方便您在项目中轻松使用。 该传感器具有±2g /±4g /±8g /±16g的用户可选全刻度,并能够以1.6 Hz至1600 Hz的输出数据速率测量加速度,它内置多种带宽的多种运行模式,您可以按需选择合适的模式。
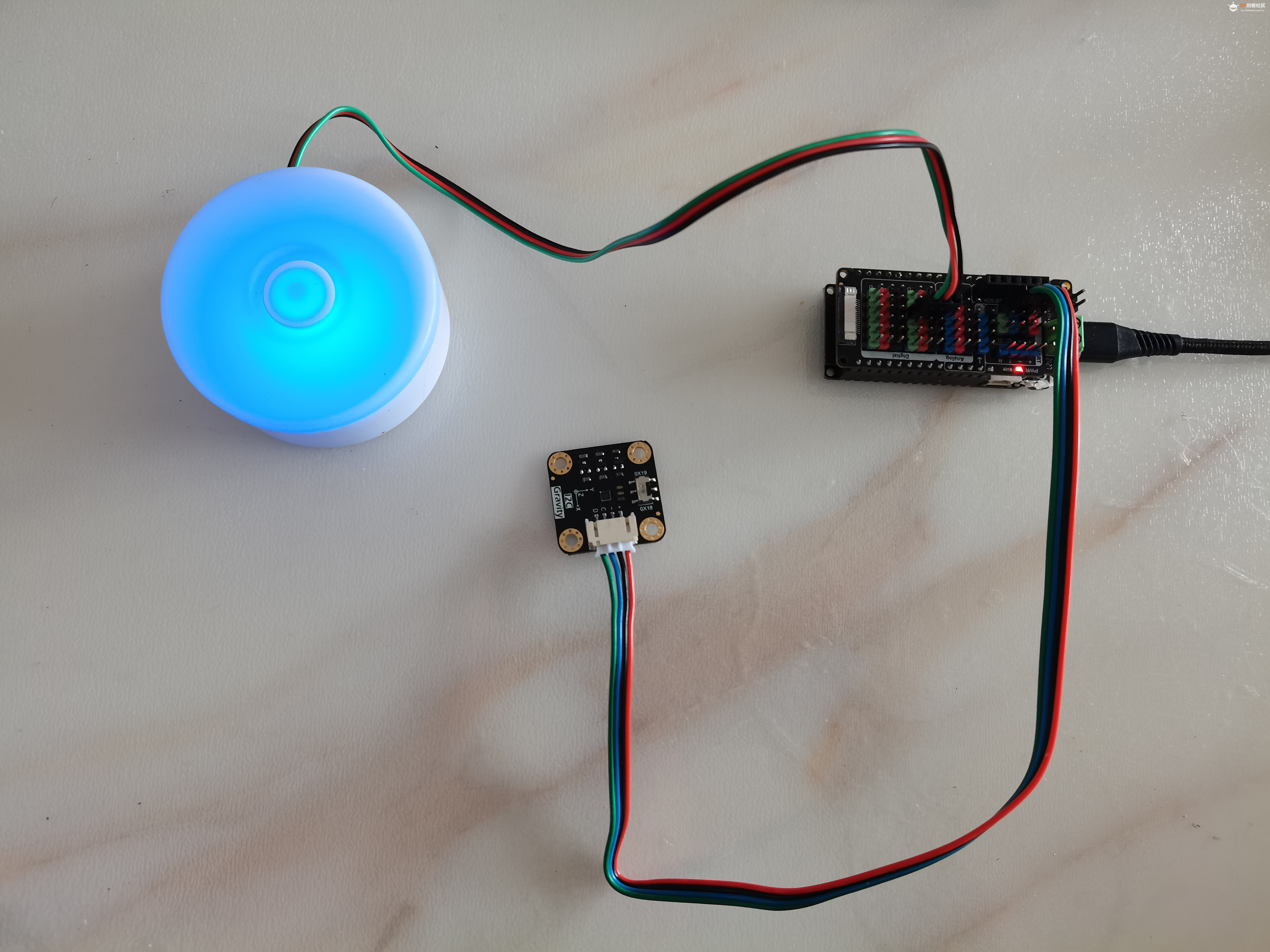
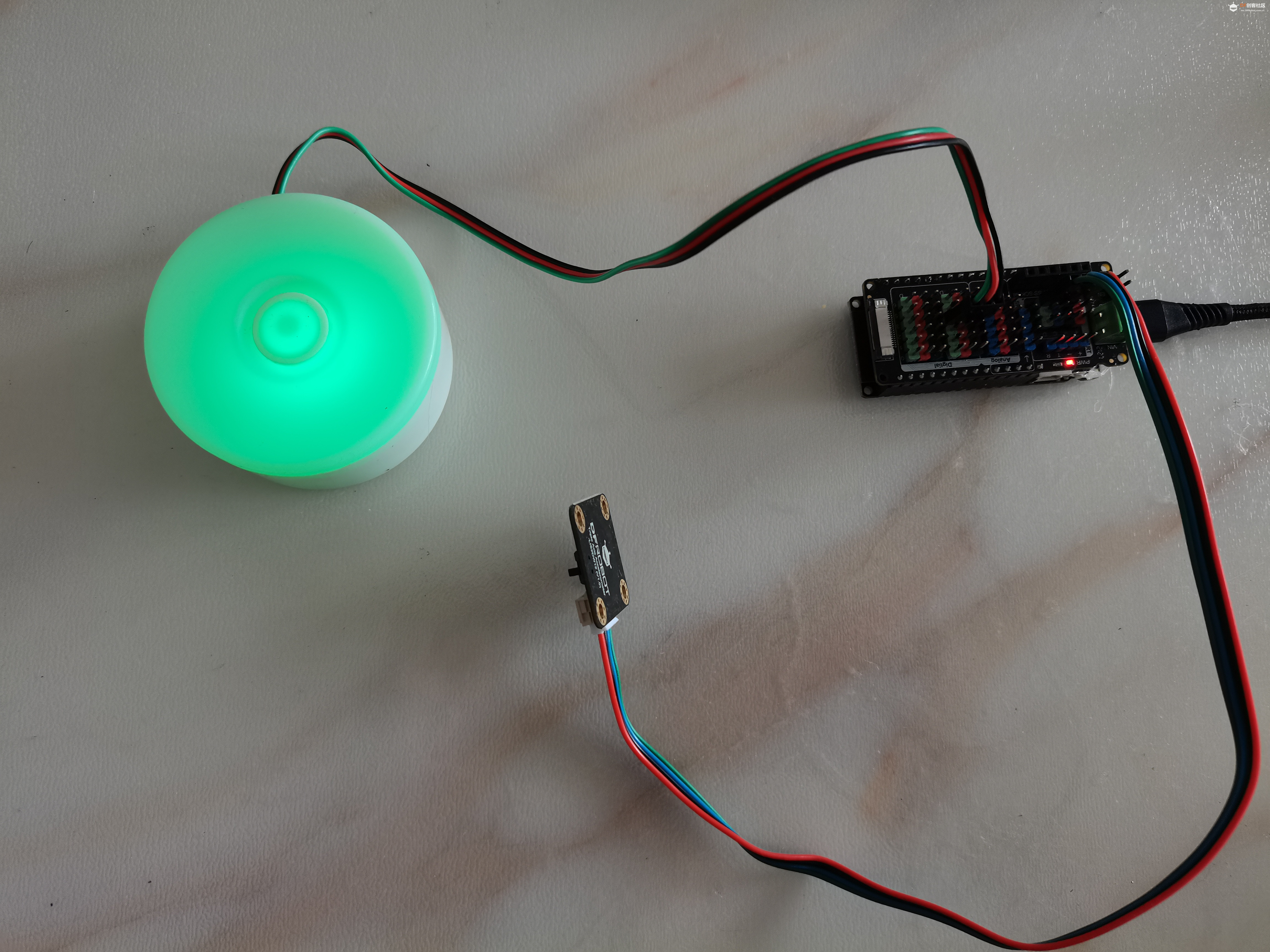
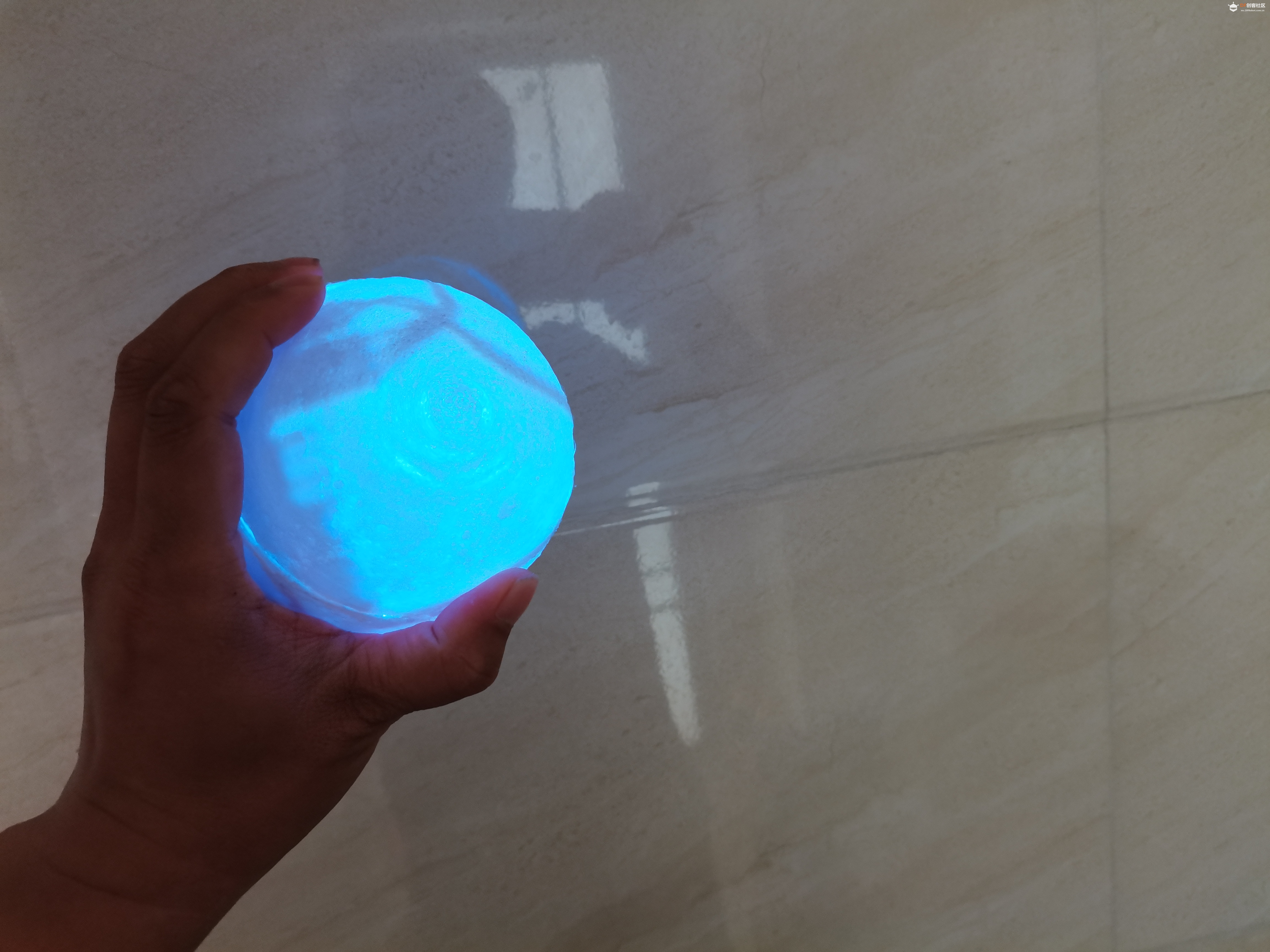
-
- /**!
- * @file orientation.ino
- * @brief When detecting the orientation of the module, the sensor can detect the following six events:
- * @n Positive z-axis is facing up
- * @n Positive z-axis is facing down
- * @n Positive y-axis is facing up
- * @n Positive y-axis is facing down
- * @n Positive x-axis is facing up
- * @n Positive x-axis is facing down
- * @n When using SPI, chip select pin can be modified by changing the value of macro LIS2DW12_CS
- * @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
- * @licence The MIT License (MIT)
- * @author [fengli](li.feng@dfrobot.com)
- * @version V1.0
- * @date 2021-01-16
- * @get from https://www.dfrobot.com
- * @https://github.com/DFRobot/DFRobot_LIS
- */
- #include <DFRobot_LIS2DW12.h>
- #include <Adafruit_NeoPixel.h>
-
- #define PIN_LED 16
- #define NUM_LED 1
-
- Adafruit_NeoPixel pixels(NUM_LED, PIN_LED, NEO_GRB + NEO_KHZ800);
-
- //When using I2C communication, use the following program to construct an object by DFRobot_LIS2DW12_I2C
- /*!
- * @brief Constructor
- * @param pWire I2c controller
- * @param addr I2C address(0x18/0x19)
- */
- DFRobot_LIS2DW12_I2C acce(&Wire,0x18);
- //DFRobot_LIS2DW12_I2C acce;
-
-
- //When using SPI communication, use the following program to construct an object by DFRobot_LIS2DW12_SPI
- #if defined(ESP32) || defined(ESP8266)
- #define LIS2DW12_CS D3
- #elif defined(__AVR__) || defined(Arduino_SAM_ZERO)
- #define LIS2DW12_CS 3
- #elif (defined NRF5)
- #define LIS2DW12_CS 2 //The pin on the development board with the corresponding silkscreen printed as P2
- #endif
- /*!
- * @brief Constructor
- * @param cs Chip selection pinChip selection pin
- * @param spi SPI controller
- */
- //DFRobot_LIS2DW12_SPI acce(/*cs = */LIS2DW12_CS,&SPI);
- //DFRobot_LIS2DW12_SPI acce(/*cs = */LIS2DW12_CS);
-
- int lastOrientation = 0; //No event happened
-
- void setup(void){
- pixels.begin();
- Serial.begin(9600);
- while(!acce.begin()){
- Serial.println("Communication failed, check the connection and I2C address setting when using I2C communication.");
- delay(1000);
- }
- Serial.print("chip id : ");
- Serial.println(acce.getID(),HEX);
- //Chip soft reset
- acce.softReset();
-
- /**!
- Set the sensor measurement range:
- e2_g /<±2g>/
- e4_g /<±4g>/
- e8_g /<±8g>/
- e16_g /< ±16g>/
- */
- acce.setRange(DFRobot_LIS2DW12::e2_g);
-
- /**!
- Set power mode:
- eHighPerformance_14bit /<High-Performance Mode,14-bit resolution>/
- eContLowPwr4_14bit /<Continuous measurement,Low-Power Mode 4(14-bit resolution)>/
- eContLowPwr3_14bit /<Continuous measurement,Low-Power Mode 3(14-bit resolution)>/
- eContLowPwr2_14bit /<Continuous measurement,Low-Power Mode 2(14-bit resolution)/
- eContLowPwr1_12bit /<Continuous measurement,Low-Power Mode 1(12-bit resolution)>/
- eSingleLowPwr4_14bit /<Single data conversion on demand mode,Low-Power Mode 4(14-bit resolution)>/
- eSingleLowPwr3_14bit /<Single data conversion on demand mode,Low-Power Mode 3(14-bit resolution)>/
- eSingleLowPwr2_14bit /<Single data conversion on demand mode,Low-Power Mode 2(14-bit resolution)>/
- eSingleLowPwr1_12bit /<Single data conversion on demand mode,Low-Power Mode 1(12-bit resolution)>/
- eHighPerformanceLowNoise_14bit /<High-Performance Mode,Low-noise enabled,14-bit resolution>/
- eContLowPwrLowNoise4_14bit /<Continuous measurement,Low-Power Mode 4(14-bit resolution,Low-noise enabled)>/
- eContLowPwrLowNoise3_14bit /<Continuous measurement,Low-Power Mode 3(14-bit resolution,Low-noise enabled)>/
- eContLowPwrLowNoise2_14bit /<Continuous measurement,Low-Power Mode 2(14-bit resolution,Low-noise enabled)>/
- eContLowPwrLowNoise1_12bit /<Continuous measurement,Low-Power Mode 1(12-bit resolution,Low-noise enabled)>/
- eSingleLowPwrLowNoise4_14bit /<Single data conversion on demand mode,Low-Power Mode 4(14-bit resolution),Low-noise enabled>/
- eSingleLowPwrLowNoise3_14bit /<Single data conversion on demand mode,Low-Power Mode 3(14-bit resolution),Low-noise enabled>/
- eSingleLowPwrLowNoise2_14bit /<Single data conversion on demand mode,Low-Power Mode 2(14-bit resolution),Low-noise enabled>/
- eSingleLowPwrLowNoise1_12bit /<Single data conversion on demand mode,Low-Power Mode 1(12-bit resolution),Low-noise enabled>/
- */
- acce.setPowerMode(DFRobot_LIS2DW12::eContLowPwrLowNoise1_12bit);
-
- /**!
- Set the sensor data collection rate:
- eRate_0hz /<Measurement off>/
- eRate_1hz6 /<1.6hz, use only under low-power mode>/
- eRate_12hz5 /<12.5hz>/
- eRate_25hz
- eRate_50hz
- eRate_100hz
- eRate_200hz
- eRate_400hz /<Use only under High-Performance mode>/
- eRate_800hz /<Use only under High-Performance mode>/
- eRate_1k6hz /<Use only under High-Performance mode>/
- eSetSwTrig /<The software triggers a single measurement>/
- */
- acce.setDataRate(DFRobot_LIS2DW12::eRate_200hz);
-
- /**!
- Set the threshold of the angle when turning:
- eDegrees80 (80°)
- eDegrees70 (70°)
- eDegrees60 (60°)
- eDegrees50 (50°)
- */
- acce.set6DThreshold(DFRobot_LIS2DW12::eDegrees60);
-
- /**!
- Set the interrupt source of the int1 pin:
- eDoubleTap(Double click)
- eFreeFall(Free fall)
- eWakeUp(wake)
- eSingleTap(single-Click)
- e6D(Orientation change check)
- */
- acce.setInt1Event(DFRobot_LIS2DW12::e6D);
-
- delay(1000);
- }
-
- void loop(void){
- //check Changes detected in six directions
- if(acce.oriChangeDetected()){
- DFRobot_LIS2DW12::eOrient_t orientation = acce.getOrientation();
- if(lastOrientation != orientation){
- if(orientation == DFRobot_LIS2DW12::eXDown){
- pixels.setPixelColor(0, pixels.Color(255,0,0));
- pixels.show();
- }
- if(orientation == DFRobot_LIS2DW12::eXUp){
- pixels.setPixelColor(0, pixels.Color(255,255,0));
- pixels.show();
- }
- if(orientation == DFRobot_LIS2DW12::eYDown){
- pixels.setPixelColor(0, pixels.Color(255,255,255));
- pixels.show();
- }
- if(orientation == DFRobot_LIS2DW12::eYUp){
- pixels.setPixelColor(0, pixels.Color(0,255,0));
- pixels.show();
- }
- if(orientation == DFRobot_LIS2DW12::eZDown){
- pixels.setPixelColor(0, pixels.Color(0,255,255));
- pixels.show();
- }
- if(orientation == DFRobot_LIS2DW12::eZUp){
- pixels.setPixelColor(0, pixels.Color(0,0,255));
- pixels.show();
- }
- lastOrientation = orientation;
- }
- }
- }
复制代码
2、演示视频
|