本帖最后由 云天 于 2022-10-2 19:52 编辑
【项目设计】
使用自发电无线开关,控制变换灯环炫彩效果。
【硬件】
主板使用Arduino,灯环为93灯/6环 WS2812B RGB 圆环灯。
【fastled库】
FastLED 是一款功能强大,简单易用的控制WS2812, LPD8806, 等LED光带的Arduino第三方库。 目前FastLED是公认的Arduino开发者应用最为广泛的LED控制库之一。下载地址:https://github.com/FastLED/FastLED/releases
【库安装】
使用“加载库”,加载库文件“FastLED-3.5.0.zip”。
【测试】
1、点亮一个灯,灯环接12引脚。
- #include <FastLED.h>
- #define NUM_LEDS 1
- #define DATA_PIN 12
- #define CLOCK_PIN 13
- CRGB leds[NUM_LEDS];
- void setup() {
- FastLED.addLeds<NEOPIXEL, DATA_PIN>(leds, NUM_LEDS); // GRB ordering is assumed
- }
- void loop() {
- leds[0] = CRGB::Red;
- FastLED.show();
- delay(500);
- leds[0] = CRGB::Black;
- FastLED.show();
- delay(500);
- }
复制代码
2、同时点亮两个灯环,两灯环分别接12,11引脚。
- #define DATA_PIN1 12
- #define DATA_PIN2 11
- void setup() {
-
- FastLED.addLeds<LED_TYPE,DATA_PIN1,COLOR_ORDER>(leds, NUM_LEDS).setCorrection(TypicalLEDStrip);
- FastLED.addLeds<LED_TYPE,DATA_PIN2,COLOR_ORDER>(leds, NUM_LEDS).setCorrection(TypicalLEDStrip);
- }
复制代码
【制作灯盒】
【程序代码】
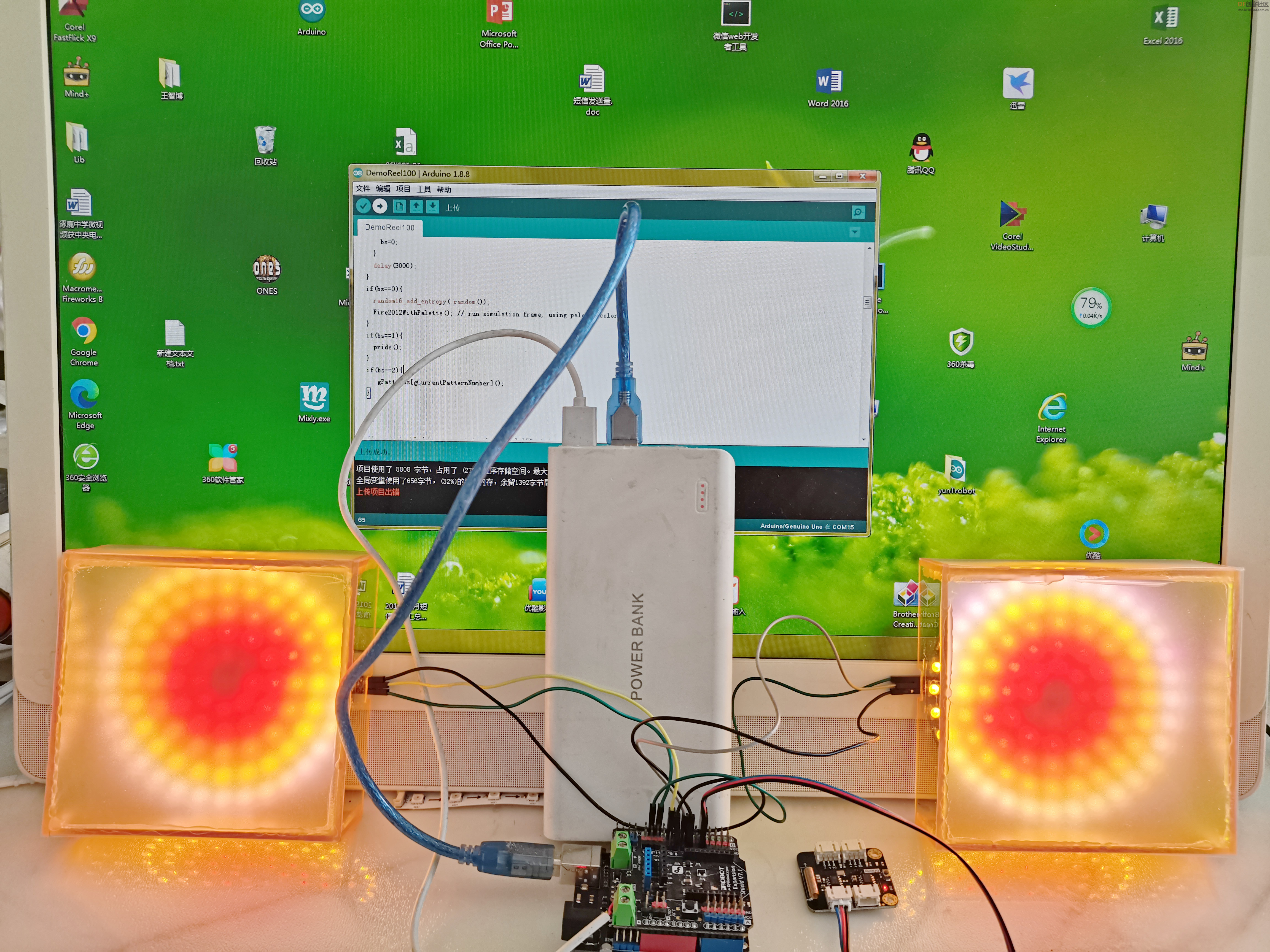
- #include <FastLED.h>
-
- FASTLED_USING_NAMESPACE
-
- #define DATA_PIN1 12
- #define DATA_PIN2 11
- //#define CLK_PIN 4
- #define LED_TYPE WS2811
- #define COLOR_ORDER GRB
- #define NUM_LEDS 93
- CRGB leds[NUM_LEDS];
-
- #define BRIGHTNESS 96
- #define FRAMES_PER_SECOND 120
- CRGBPalette16 gPal;
- bool gReverseDirection = false;
- int bs;
- void setup() {
- bs=0;
- delay(3000); // 3 second delay for recovery
- gPal = HeatColors_p;
-
- FastLED.addLeds<LED_TYPE,DATA_PIN1,COLOR_ORDER>(leds, NUM_LEDS).setCorrection(TypicalLEDStrip);
- FastLED.addLeds<LED_TYPE,DATA_PIN2,COLOR_ORDER>(leds, NUM_LEDS).setCorrection(TypicalLEDStrip);
-
- FastLED.setBrightness(BRIGHTNESS);
- }
-
- typedef void (*SimplePatternList[])();
- SimplePatternList gPatterns = { rainbow, rainbowWithGlitter, confetti, sinelon, juggle, bpm };
-
- uint8_t gCurrentPatternNumber = 0; // Index number of which pattern is current
- uint8_t gHue = 0; // rotating "base color" used by many of the patterns
-
- void loop()
- {
- if (digitalRead(4)) {
- bs += 1;
- if(bs==3){
- bs=0;
- }
- delay(3000);
- }
- if(bs==0){
- random16_add_entropy( random());
- Fire2012WithPalette(); // run simulation frame, using palette colors
- }
- if(bs==1){
- pride();
- }
- if(bs==2){
- gPatterns[gCurrentPatternNumber]();
- }
-
- FastLED.show();
-
- FastLED.delay(1000/FRAMES_PER_SECOND);
-
-
- EVERY_N_MILLISECONDS( 20 ) { gHue++; } // slowly cycle the "base color" through the rainbow
- EVERY_N_SECONDS( 10 ) { nextPattern(); } // change patterns periodically
- }
-
- #define ARRAY_SIZE(A) (sizeof(A) / sizeof((A)[0]))
-
- void nextPattern()
- {
-
- gCurrentPatternNumber = (gCurrentPatternNumber + 1) % ARRAY_SIZE( gPatterns);
- }
-
- void rainbow()
- {
-
- fill_rainbow( leds, NUM_LEDS, gHue, 7);
- }
-
- void rainbowWithGlitter()
- {
-
- rainbow();
- addGlitter(80);
- }
-
- void addGlitter( fract8 chanceOfGlitter)
- {
- if( random8() < chanceOfGlitter) {
- leds[ random16(NUM_LEDS) ] += CRGB::White;
- }
- }
-
- void confetti()
- {
-
- fadeToBlackBy( leds, NUM_LEDS, 10);
- int pos = random16(NUM_LEDS);
- leds[pos] += CHSV( gHue + random8(64), 200, 255);
- }
-
- void sinelon()
- {
-
- fadeToBlackBy( leds, NUM_LEDS, 20);
- int pos = beatsin16( 13, 0, NUM_LEDS-1 );
- leds[pos] += CHSV( gHue, 255, 192);
- }
-
- void bpm()
- {
-
- uint8_t BeatsPerMinute = 62;
- CRGBPalette16 palette = PartyColors_p;
- uint8_t beat = beatsin8( BeatsPerMinute, 64, 255);
- for( int i = 0; i < NUM_LEDS; i++) { //9948
- leds[i] = ColorFromPalette(palette, gHue+(i*2), beat-gHue+(i*10));
- }
- }
-
- void juggle() {
-
- fadeToBlackBy( leds, NUM_LEDS, 20);
- uint8_t dothue = 0;
- for( int i = 0; i < 8; i++) {
- leds[beatsin16( i+7, 0, NUM_LEDS-1 )] |= CHSV(dothue, 200, 255);
- dothue += 32;
- }
- }
- void pride()
- {
- static uint16_t sPseudotime = 0;
- static uint16_t sLastMillis = 0;
- static uint16_t sHue16 = 0;
-
- uint8_t sat8 = beatsin88( 87, 220, 250);
- uint8_t brightdepth = beatsin88( 341, 96, 224);
- uint16_t brightnessthetainc16 = beatsin88( 203, (25 * 256), (40 * 256));
- uint8_t msmultiplier = beatsin88(147, 23, 60);
-
- uint16_t hue16 = sHue16;//gHue * 256;
- uint16_t hueinc16 = beatsin88(113, 1, 3000);
-
- uint16_t ms = millis();
- uint16_t deltams = ms - sLastMillis ;
- sLastMillis = ms;
- sPseudotime += deltams * msmultiplier;
- sHue16 += deltams * beatsin88( 400, 5,9);
- uint16_t brightnesstheta16 = sPseudotime;
-
- for( uint16_t i = 0 ; i < NUM_LEDS; i++) {
- hue16 += hueinc16;
- uint8_t hue8 = hue16 / 256;
-
- brightnesstheta16 += brightnessthetainc16;
- uint16_t b16 = sin16( brightnesstheta16 ) + 32768;
-
- uint16_t bri16 = (uint32_t)((uint32_t)b16 * (uint32_t)b16) / 65536;
- uint8_t bri8 = (uint32_t)(((uint32_t)bri16) * brightdepth) / 65536;
- bri8 += (255 - brightdepth);
-
- CRGB newcolor = CHSV( hue8, sat8, bri8);
-
- uint16_t pixelnumber = i;
- pixelnumber = (NUM_LEDS-1) - pixelnumber;
-
- nblend( leds[pixelnumber], newcolor, 64);
- }
- }
- #define COOLING 55
-
- #define SPARKING 120
-
-
- void Fire2012WithPalette()
- {
-
- static uint8_t heat[NUM_LEDS];
-
-
- for( int i = 0; i < NUM_LEDS; i++) {
- heat[i] = qsub8( heat[i], random8(0, ((COOLING * 10) / NUM_LEDS) + 2));
- }
-
-
- for( int k= NUM_LEDS - 1; k >= 2; k--) {
- heat[k] = (heat[k - 1] + heat[k - 2] + heat[k - 2] ) / 3;
- }
-
-
- if( random8() < SPARKING ) {
- int y = random8(7);
- heat[y] = qadd8( heat[y], random8(160,255) );
- }
-
-
- for( int j = 0; j < NUM_LEDS; j++) {
-
- uint8_t colorindex = scale8( heat[j], 240);
- CRGB color = ColorFromPalette( gPal, colorindex);
- int pixelnumber;
- if( gReverseDirection ) {
- pixelnumber = (NUM_LEDS-1) - j;
- } else {
- pixelnumber = j;
- }
- leds[pixelnumber] = color;
- }
- }
复制代码
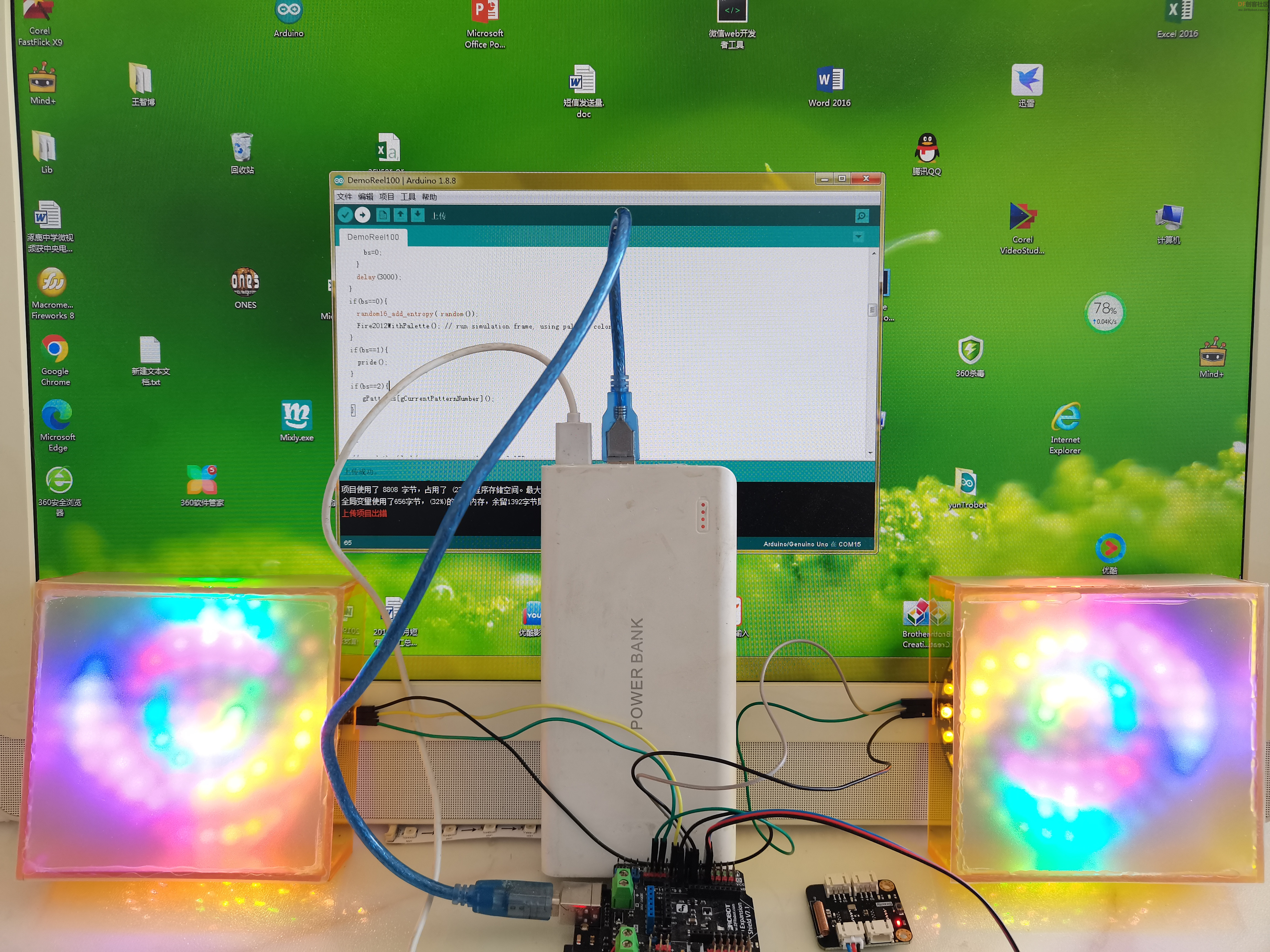
【演示视频】
|