本帖最后由 云天 于 2023-9-17 13:31 编辑
【项目背景】
最近在DF商城上看到“空中飞鼠键盘”,它连到行空板上时,您不需安装任何驱动,只需把它插上去,就能立即使用了。它使用了2.4G无线传输技术,传输距离可达30米。无论是在客厅还是办公室,您都可使用它进行远程遥控。
【项目设计】
使用行空板结合“空中飞鼠键盘”,利用“PYgame库”,制作多个使用键盘控制的行空板小游戏。
俄罗斯方块
贪吃蛇
飞机大战
【演示视频】
【飞机大战】
这次用Mind+软件Python模式下,连接终端行空板,利用Python中的pygame模块来完成一个飞机大战的小游戏;基本思路是通过方向键来控制飞机的左右移动射击飞船。
创建一个可以左右移动的小飞机,用户可以通过空格space键来控制飞机发射子弹
1.创建背景,加载角色
- import pygame
- import random
- import time
- # 定义窗口的大小
- WINDOW_WIDTH = 240
- WINDOW_HEIGHT = 320
-
- # 初始化 Pygame
- pygame.init()
-
- # 创建窗口 设置屏幕的分辨率
- window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
- pygame.display.set_caption('Plane Fight')
-
- # 加载角色图片
- background_image = pygame.image.load('background.png')
- player_image = pygame.image.load('player.png')
- enemy_image = pygame.image.load('enemy.png')
- bullet_image = pygame.image.load('bullet.png')
-
- # 定义颜色
- BLACK = (0, 0, 0)
- WHITE = (255, 255, 255)
- RED = (255, 0, 0)
-
- # 定义字体
- font = pygame.font.Font(None, 36)
复制代码
2.主循环体中,实现键盘控制
-
- while not game_over:
- # 处理事件
- for event in pygame.event.get():
- if event.type == pygame.QUIT:
- game_over = True
-
- elif event.type == pygame.KEYDOWN:
- if event.key == pygame.K_RIGHT:
- # 当用户按下键位时标志位为True
- player.mv_right = True
- elif event.key == pygame.K_LEFT:
- player.mv_left = True
- if event.key == pygame.K_SPACE:#用户按下空格之后会创建一个子弹
-
- player.mv_shoot = True
-
-
- elif event.type == pygame.KEYUP:
- if event.key == pygame.K_RIGHT:
- # 当用户松开键位为false
- player.mv_right = False
- elif event.key == pygame.K_LEFT:
- player.mv_left = False
- if event.key == pygame.K_SPACE:
-
- player.mv_shoot = False
-
复制代码
3.绘制小飞机玩家
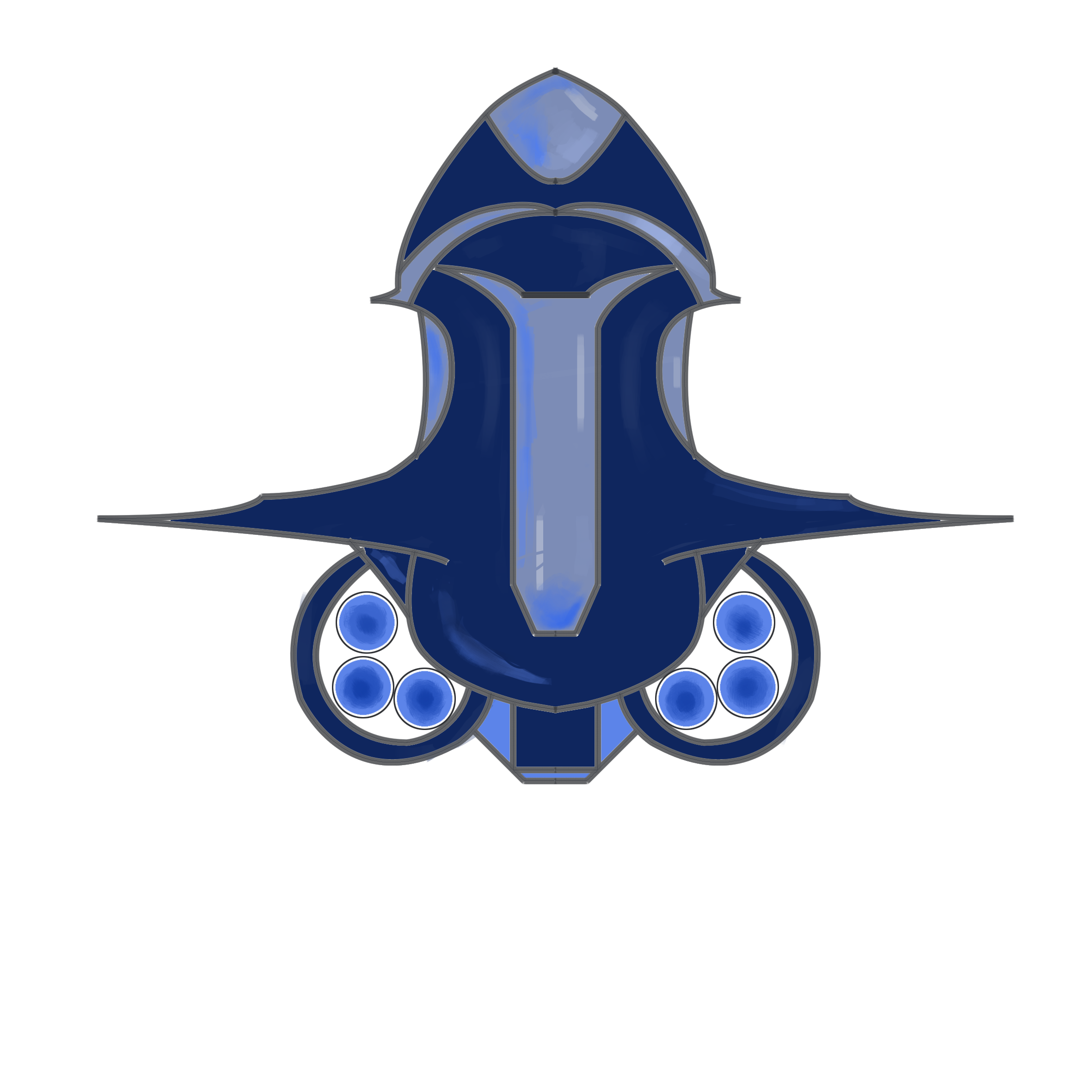
定义一个Player类,来存储飞机的各种行为。
-
- # 定义玩家类
- class Player(pygame.sprite.Sprite):
- def __init__(self):
- super().__init__()
-
- self.image = player_image
- self.rect = self.image.get_rect()
- self.rect.centerx = WINDOW_WIDTH // 2
- self.rect.bottom = WINDOW_HEIGHT - 10
- self.speed = 5
- self.mv_right = False
- self.mv_left = False
- self.mv_shoot= False
- self.Bullet_num=10
- self.shoot_speed=1
- self.last_shoot_time=time.time()
- # 移动
- # 定义一个调整小飞机位置的方法
- def update(self):
- # 根据标志位的调整小飞机的位置
- #实现左右移动,控制小飞机持续移动
- if self.mv_right:
- self.rect.centerx += self.speed
- if self.mv_left:
- self.rect.centerx -= self.speed
- if self.rect.left < 0:
- self.rect.left = 0
- elif self.rect.right > WINDOW_WIDTH:#限制小飞机的活动范围
- self.rect.right = WINDOW_WIDTH
- if self.mv_shoot:
- curTime_shoot = time.time()
- if curTime_shoot-self.last_shoot_time>self.shoot_speed:#限制子弹发射频率
- self.last_shoot_time = time.time()
- player.shoot()
- # 射击
- def shoot(self):
- bullet = Bullet(self.rect.centerx, self.rect.top)
- all_sprites.add(bullet)
- bullets.add(bullet)
复制代码
-
- # 创建精灵组
- all_sprites = pygame.sprite.Group()
- enemies = pygame.sprite.Group()
- bullets = pygame.sprite.Group()
-
- # 创建玩家并添加到精灵组
- player = Player()
- all_sprites.add(player)
复制代码
4.完成射击功能
通过玩家按下空格来发射子弹
-
- # 定义子弹类
- class Bullet(pygame.sprite.Sprite):
- def __init__(self, x, y):
- super().__init__()
- self.image = bullet_image
- self.rect = self.image.get_rect()
- self.rect.centerx = x
- self.rect.bottom = y
- self.speed = -10
-
- # 移动
- def update(self):
- self.rect.y += self.speed
- if self.rect.bottom < 0:#删除已经消失的子弹
- self.kill()
复制代码
5.制作飞船
现在小飞机也创建完成了,现在就该创建小飞机的敌人了,同样通过一个类来控制其所有行为。
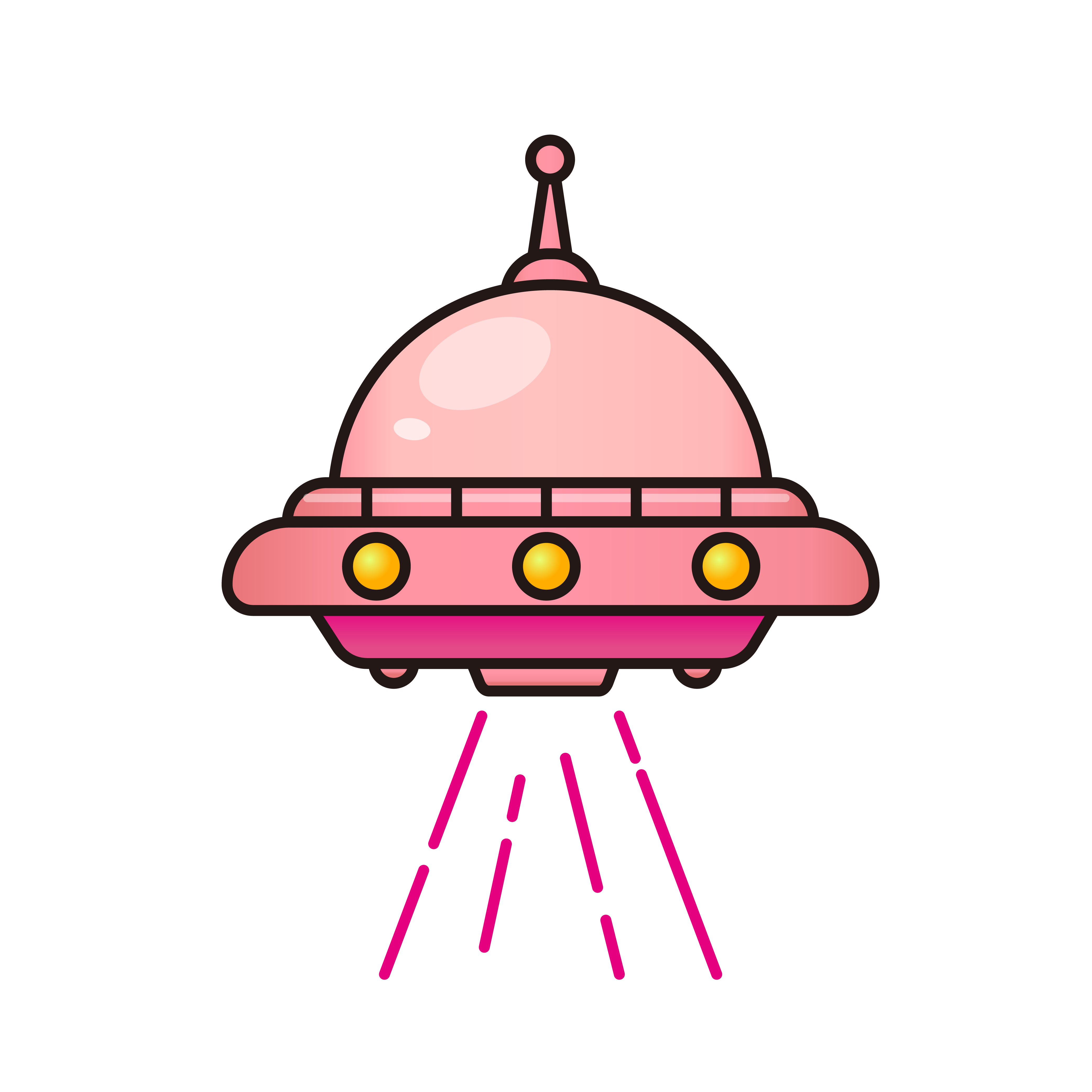
-
- # 定义敌机类
- class Enemy(pygame.sprite.Sprite):
- def __init__(self):
- super().__init__()
- self.image = enemy_image
- self.rect = self.image.get_rect()
- self.rect.x = random.randint(0, WINDOW_WIDTH - self.rect.width)
- self.rect.y = random.randint(-100, -self.rect.height)
- self.speed = random.randint(1, 2)
-
- # 移动
- def update(self):
- self.rect.y += self.speed
- if self.rect.top > WINDOW_HEIGHT:
- self.kill()
-
复制代码
玩家小飞机可以射杀飞船、当飞船碰到小飞机GAMEOVER,飞船碰到地面也GAMEOVER
-
- # 生成敌机
- curTime = time.time()
-
- if len(enemies) < 10 and curTime - last_move_time>random.randint(3,enemy_speed+3):
- print(enemy_speed)
- last_move_time=time.time()
- enemy = Enemy()
- all_sprites.add(enemy)
- enemies.add(enemy)
-
- # 检测子弹是否击中敌机
- hits = pygame.sprite.groupcollide(enemies, bullets, True, True)
- for hit in hits:
- score += 10
- #随得分增加,加大敌机生成频率和生成子弹频率
- if score<1000:
- enemy_speed=10-int(score/100)
- player.shoot_speed=1-int(score/100)/10
- else:
- enemy_speed=1
- player.shoot_speed=0.1
- # 检测敌机是否撞击玩家
- hits = pygame.sprite.spritecollide(player, enemies, False)
- if len(hits) > 0:
- game_over = True
复制代码
6.更新角色游戏状态
-
- # 更新游戏状态
- all_sprites.update()
- # 清屏
- window.fill(BLACK)
-
- # 绘制背景
- window.blit(background_image, (0, 0))
-
- # 绘制精灵组中的所有元素
- all_sprites.draw(window)
-
- # 显示得分
- score_text = font.render(f'Score: {score}', True, WHITE)
- window.blit(score_text, (10, 10))
-
- # 更新屏幕
- pygame.display.flip()
-
- # 控制帧率
- clock.tick(60)
复制代码
7.游戏结束
-
-
- # 游戏结束,显示最终得分
- game_over_text = font.render('Game Over', True, RED)
- score_text = font.render(f'Final Score: {score}', True, WHITE)
- window.blit(game_over_text, (WINDOW_WIDTH // 2 - game_over_text.get_width() // 2, WINDOW_HEIGHT // 2 - game_over_text.get_height() // 2 - 30))
- window.blit(score_text, (WINDOW_WIDTH // 2 - score_text.get_width() // 2, WINDOW_HEIGHT // 2 - score_text.get_height() // 2 + 30))
- pygame.display.flip()
-
- # 等待 10 秒后关闭窗口
- pygame.time.wait(10000)
- pygame.quit()
复制代码
8.完整代码
-
-
- import pygame
- import random
- import time
- # 定义窗口的大小
- WINDOW_WIDTH = 240
- WINDOW_HEIGHT = 320
-
- # 初始化 Pygame
- pygame.init()
-
- # 创建窗口 设置屏幕的分辨率
- window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
- pygame.display.set_caption('Plane Fight')
-
- # 加载角色图片
- background_image = pygame.image.load('background.png')
- player_image = pygame.image.load('player.png')
- enemy_image = pygame.image.load('enemy.png')
- bullet_image = pygame.image.load('bullet.png')
-
- # 定义颜色
- BLACK = (0, 0, 0)
- WHITE = (255, 255, 255)
- RED = (255, 0, 0)
-
- # 定义字体
- font = pygame.font.Font(None, 36)
-
- # 定义玩家类
- class Player(pygame.sprite.Sprite):
- def __init__(self):
- super().__init__()
-
- self.image = player_image
- self.rect = self.image.get_rect()
- self.rect.centerx = WINDOW_WIDTH // 2
- self.rect.bottom = WINDOW_HEIGHT - 10
- self.speed = 5
- self.mv_right = False
- self.mv_left = False
- self.mv_shoot= False
- self.Bullet_num=10
- self.shoot_speed=1
- self.last_shoot_time=time.time()
- # 移动
- # 定义一个调整小飞机位置的方法
- def update(self):
- # 根据标志位的调整小飞机的位置
-
- if self.mv_right:
- self.rect.centerx += self.speed
- if self.mv_left:
- self.rect.centerx -= self.speed
- if self.rect.left < 0:
- self.rect.left = 0
- elif self.rect.right > WINDOW_WIDTH:
- self.rect.right = WINDOW_WIDTH
- if self.mv_shoot:
- curTime_shoot = time.time()
- if curTime_shoot-self.last_shoot_time>self.shoot_speed:
- self.last_shoot_time = time.time()
- player.shoot()
- # 射击
- def shoot(self):
- bullet = Bullet(self.rect.centerx, self.rect.top)
- all_sprites.add(bullet)
- bullets.add(bullet)
-
- # 定义敌机类
- class Enemy(pygame.sprite.Sprite):
- def __init__(self):
- super().__init__()
- self.image = enemy_image
- self.rect = self.image.get_rect()
- self.rect.x = random.randint(0, WINDOW_WIDTH - self.rect.width)
- self.rect.y = random.randint(-100, -self.rect.height)
- self.speed = random.randint(1, 2)
-
- # 移动
- def update(self):
- self.rect.y += self.speed
- if self.rect.top > WINDOW_HEIGHT:
- self.kill()
-
- # 定义子弹类
- class Bullet(pygame.sprite.Sprite):
- def __init__(self, x, y):
- super().__init__()
- self.image = bullet_image
- self.rect = self.image.get_rect()
- self.rect.centerx = x
- self.rect.bottom = y
- self.speed = -10
-
- # 移动
- def update(self):
- self.rect.y += self.speed
- if self.rect.bottom < 0:
- self.kill()
-
- # 创建精灵组
- all_sprites = pygame.sprite.Group()
- enemies = pygame.sprite.Group()
- bullets = pygame.sprite.Group()
-
- # 创建玩家并添加到精灵组
- player = Player()
- all_sprites.add(player)
-
- # 定义游戏循环
- clock = pygame.time.Clock()
- score = 0
- game_over = False
- last_move_time=time.time()
-
- enemy_speed=10
- while not game_over:
- # 处理事件
- for event in pygame.event.get():
- if event.type == pygame.QUIT:
- game_over = True
-
- elif event.type == pygame.KEYDOWN:
- if event.key == pygame.K_RIGHT:
- # 当用户按下键位时标志位为True
- player.mv_right = True
- elif event.key == pygame.K_LEFT:
- player.mv_left = True
- if event.key == pygame.K_SPACE:
-
- player.mv_shoot = True
-
-
- elif event.type == pygame.KEYUP:
- if event.key == pygame.K_RIGHT:
- # 当用户松开键位为false
- player.mv_right = False
- elif event.key == pygame.K_LEFT:
- player.mv_left = False
- if event.key == pygame.K_SPACE:
-
- player.mv_shoot = False
-
-
- # 更新游戏状态
- all_sprites.update()
-
- # 生成敌机
- curTime = time.time()
-
- if len(enemies) < 10 and curTime - last_move_time>random.randint(3,enemy_speed+3):
- print(enemy_speed)
- last_move_time=time.time()
- enemy = Enemy()
- all_sprites.add(enemy)
- enemies.add(enemy)
-
- # 检测子弹是否击中敌机
- hits = pygame.sprite.groupcollide(enemies, bullets, True, True)
- for hit in hits:
- score += 10
- #随得分增加,加大敌机生成频率和生成子弹频率
- if score<1000:
- enemy_speed=10-int(score/100)
- player.shoot_speed=1-int(score/100)/10
- else:
- enemy_speed=1
- player.shoot_speed=0.1
- # 检测敌机是否撞击玩家
- hits = pygame.sprite.spritecollide(player, enemies, False)
- if len(hits) > 0:
- game_over = True
-
- # 清屏
- window.fill(BLACK)
-
- # 绘制背景
- window.blit(background_image, (0, 0))
-
- # 绘制精灵组中的所有元素
- all_sprites.draw(window)
-
- # 显示得分
- score_text = font.render(f'Score: {score}', True, WHITE)
- window.blit(score_text, (10, 10))
-
- # 更新屏幕
- pygame.display.flip()
-
- # 控制帧率
- clock.tick(60)
-
- # 游戏结束,显示最终得分
- game_over_text = font.render('Game Over', True, RED)
- score_text = font.render(f'Final Score: {score}', True, WHITE)
- window.blit(game_over_text, (WINDOW_WIDTH // 2 - game_over_text.get_width() // 2, WINDOW_HEIGHT // 2 - game_over_text.get_height() // 2 - 30))
- window.blit(score_text, (WINDOW_WIDTH // 2 - score_text.get_width() // 2, WINDOW_HEIGHT // 2 - score_text.get_height() // 2 + 30))
- pygame.display.flip()
-
- # 等待 3 秒后关闭窗口
- pygame.time.wait(10000)
- pygame.quit()
-
复制代码
【俄罗斯方块】
-
- import sys
- import time
- import pygame
- from pygame.locals import *
- import blocks
-
- SIZE = 10 # 每个小方格大小
- BLOCK_HEIGHT = 32 # 游戏区高度
- BLOCK_WIDTH = 20 # 游戏区宽度
- BORDER_WIDTH = 4 # 游戏区边框宽度
- BORDER_COLOR = (40, 40, 200) # 游戏区边框颜色
- SCREEN_WIDTH = SIZE * (BLOCK_WIDTH + 4) # 游戏屏幕的宽
- SCREEN_HEIGHT = SIZE * BLOCK_HEIGHT # 游戏屏幕的高
- BG_COLOR = (40, 40, 60) # 背景色
- BLOCK_COLOR = (20, 128, 200) #
- BLACK = (0, 0, 0)
- RED = (200, 30, 30) # GAME OVER 的字体颜色
-
-
- def print_text(screen, font, x, y, text, fcolor=(255, 255, 255)):
- imgText = font.render(text, True, fcolor)
- screen.blit(imgText, (x, y))
-
-
- def main():
- pygame.init()
- screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
- pygame.display.set_caption('俄罗斯方块')
-
- font1 = pygame.font.SysFont('SimHei', 14) # 黑体24
- font2 = pygame.font.Font(None, 55) # GAME OVER 的字体
- font_pos_x = BLOCK_WIDTH * SIZE + BORDER_WIDTH + 2 # 右侧信息显示区域字体位置的X坐标
- gameover_size = font2.size('GAME OVER')
- font1_height = int(font1.size('score')[1])
-
- cur_block = None # 当前下落方块
- next_block = None # 下一个方块
- cur_pos_x, cur_pos_y = 0, 0
-
- game_area = None # 整个游戏区域
- game_over = True
- start = False # 是否开始,当start = True,game_over = True 时,才显示 GAME OVER
- score = 0 # 得分
- orispeed = 0.5 # 原始速度
- speed = orispeed # 当前速度
- pause = False # 暂停
- last_drop_time = None # 上次下落时间
- last_press_time = None # 上次按键时间
-
- def _dock():
- nonlocal cur_block, next_block, game_area, cur_pos_x, cur_pos_y, game_over, score, speed
- for _i in range(cur_block.start_pos.Y, cur_block.end_pos.Y + 1):
- for _j in range(cur_block.start_pos.X, cur_block.end_pos.X + 1):
- if cur_block.template[_i][_j] != '.':
- game_area[cur_pos_y + _i][cur_pos_x + _j] = '0'
- if cur_pos_y + cur_block.start_pos.Y <= 0:
- game_over = True
- else:
- # 计算消除
- remove_idxs = []
- for _i in range(cur_block.start_pos.Y, cur_block.end_pos.Y + 1):
- if all(_x == '0' for _x in game_area[cur_pos_y + _i]):
- remove_idxs.append(cur_pos_y + _i)
- if remove_idxs:
- # 计算得分
- remove_count = len(remove_idxs)
- if remove_count == 1:
- score += 100
- elif remove_count == 2:
- score += 300
- elif remove_count == 3:
- score += 700
- elif remove_count == 4:
- score += 1500
- speed = orispeed - 0.03 * (score // 10000)
- # 消除
- _i = _j = remove_idxs[-1]
- while _i >= 0:
- while _j in remove_idxs:
- _j -= 1
- if _j < 0:
- game_area[_i] = ['.'] * BLOCK_WIDTH
- else:
- game_area[_i] = game_area[_j]
- _i -= 1
- _j -= 1
- cur_block = next_block
- next_block = blocks.get_block()
- cur_pos_x, cur_pos_y = (BLOCK_WIDTH - cur_block.end_pos.X - 1) // 2, -1 - cur_block.end_pos.Y
-
- def _judge(pos_x, pos_y, block):
- nonlocal game_area
- for _i in range(block.start_pos.Y, block.end_pos.Y + 1):
- if pos_y + block.end_pos.Y >= BLOCK_HEIGHT:
- return False
- for _j in range(block.start_pos.X, block.end_pos.X + 1):
- if pos_y + _i >= 0 and block.template[_i][_j] != '.' and game_area[pos_y + _i][pos_x + _j] != '.':
- return False
- return True
-
- while True:
- for event in pygame.event.get():
- if event.type == QUIT:
- sys.exit()
- elif event.type == KEYDOWN:
- if event.key == K_RETURN:
- if game_over:
- start = True
- game_over = False
- score = 0
- last_drop_time = time.time()
- last_press_time = time.time()
- game_area = [['.'] * BLOCK_WIDTH for _ in range(BLOCK_HEIGHT)]
- cur_block = blocks.get_block()
- next_block = blocks.get_block()
- cur_pos_x, cur_pos_y = (BLOCK_WIDTH - cur_block.end_pos.X - 1) // 2, -1 - cur_block.end_pos.Y
- elif event.key == K_SPACE:
- if not game_over:
- pause = not pause
- elif event.key in (K_w, K_UP):
-
- if 0 <= cur_pos_x <= BLOCK_WIDTH - len(cur_block.template[0]):
- _next_block = blocks.get_next_block(cur_block)
- if _judge(cur_pos_x, cur_pos_y, _next_block):
- cur_block = _next_block
- elif event.key in (K_a, K_LEFT):
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- if cur_pos_x > - cur_block.start_pos.X:
- if _judge(cur_pos_x - 1, cur_pos_y, cur_block):
- cur_pos_x -= 1
- elif event.key in (K_d, K_RIGHT):
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- # 不能移除右边框
- if cur_pos_x + cur_block.end_pos.X + 1 < BLOCK_WIDTH:
- if _judge(cur_pos_x + 1, cur_pos_y, cur_block):
- cur_pos_x += 1
- elif event.key in (K_s, K_DOWN):
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- if not _judge(cur_pos_x, cur_pos_y + 1, cur_block):
- _dock()
- else:
- last_drop_time = time.time()
- cur_pos_y += 1
-
- if event.type == pygame.KEYDOWN:
- if event.key == pygame.K_LEFT:
- if not game_over and not pause:
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- if cur_pos_x > - cur_block.start_pos.X:
- if _judge(cur_pos_x - 1, cur_pos_y, cur_block):
- cur_pos_x -= 1
- if event.key == pygame.K_RIGHT:
- if not game_over and not pause:
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- # 不能移除右边框
- if cur_pos_x + cur_block.end_pos.X + 1 < BLOCK_WIDTH:
- if _judge(cur_pos_x + 1, cur_pos_y, cur_block):
- cur_pos_x += 1
- if event.key == pygame.K_DOWN:
- if not game_over and not pause:
- if time.time() - last_press_time > 0.1:
- last_press_time = time.time()
- if not _judge(cur_pos_x, cur_pos_y + 1, cur_block):
- _dock()
- else:
- last_drop_time = time.time()
- cur_pos_y += 1
-
- _draw_background(screen)
-
- _draw_game_area(screen, game_area)
-
- _draw_gridlines(screen)
-
- _draw_info(screen, font1, font_pos_x, font1_height, score)
- # 画显示信息中的下一个方块
- _draw_block(screen, next_block, font_pos_x, 30 + (font1_height + 6) * 5, 0, 0)
-
- if not game_over:
- cur_drop_time = time.time()
- if cur_drop_time - last_drop_time > speed:
- if not pause:
-
- if not _judge(cur_pos_x, cur_pos_y + 1, cur_block):
- _dock()
- else:
- last_drop_time = cur_drop_time
- cur_pos_y += 1
- else:
- if start:
- print_text(screen, font2,
- (SCREEN_WIDTH - gameover_size[0]) // 2, (SCREEN_HEIGHT - gameover_size[1]) // 2,
- 'GAME OVER', RED)
-
- # 画当前下落方块
- _draw_block(screen, cur_block, 0, 0, cur_pos_x, cur_pos_y)
-
- pygame.display.flip()
-
-
- # 画背景
- def _draw_background(screen):
- # 填充背景色
- screen.fill(BG_COLOR)
- # 画游戏区域分隔线
- pygame.draw.line(screen, BORDER_COLOR,
- (SIZE * BLOCK_WIDTH + BORDER_WIDTH // 2, 0),
- (SIZE * BLOCK_WIDTH + BORDER_WIDTH // 2, SCREEN_HEIGHT), BORDER_WIDTH)
-
-
- # 画网格线
- def _draw_gridlines(screen):
- # 画网格线 竖线
- for x in range(BLOCK_WIDTH):
- pygame.draw.line(screen, BLACK, (x * SIZE, 0), (x * SIZE, SCREEN_HEIGHT), 1)
- # 画网格线 横线
- for y in range(BLOCK_HEIGHT):
- pygame.draw.line(screen, BLACK, (0, y * SIZE), (BLOCK_WIDTH * SIZE, y * SIZE), 1)
-
-
- # 画已经落下的方块
- def _draw_game_area(screen, game_area):
- if game_area:
- for i, row in enumerate(game_area):
- for j, cell in enumerate(row):
- if cell != '.':
- pygame.draw.rect(screen, BLOCK_COLOR, (j * SIZE, i * SIZE, SIZE, SIZE), 0)
-
-
- # 画单个方块
- def _draw_block(screen, block, offset_x, offset_y, pos_x, pos_y):
- if block:
- for i in range(block.start_pos.Y, block.end_pos.Y + 1):
- for j in range(block.start_pos.X, block.end_pos.X + 1):
- if block.template[i][j] != '.':
- pygame.draw.rect(screen, BLOCK_COLOR,
- (offset_x + (pos_x + j) * SIZE, offset_y + (pos_y + i) * SIZE, SIZE, SIZE), 0)
-
-
- # 画得分等信息
- def _draw_info(screen, font, pos_x, font_height, score):
- print_text(screen, font, pos_x, 10, f'score: ')
- print_text(screen, font, pos_x, 10 + font_height + 6, f'{score}')
- print_text(screen, font, pos_x, 20 + (font_height + 6) * 2, f'speed:')
- print_text(screen, font, pos_x, 20 + (font_height + 6) * 3, f'{score // 10000}')
- print_text(screen, font, pos_x, 30 + (font_height + 6) * 4, f'next:')
-
-
- if __name__ == '__main__':
- main()
-
复制代码
【贪吃蛇】
-
- import random
- import sys
- import time
- import pygame
- from pygame.locals import *
- from collections import deque
-
- SCREEN_WIDTH = 240 # 屏幕宽度
- SCREEN_HEIGHT = 320 # 屏幕高度
- SIZE = 20 # 小方格大小
- LINE_WIDTH = 1 # 网格线宽度
-
- # 游戏区域的坐标范围
- SCOPE_X = (0, SCREEN_WIDTH // SIZE - 1)
- SCOPE_Y = (2, SCREEN_HEIGHT // SIZE - 1)
-
- # 食物的分值及颜色
- FOOD_STYLE_LIST = [(10, (255, 100, 100)), (20, (100, 255, 100)), (30, (100, 100, 255))]
-
- LIGHT = (100, 100, 100)
- DARK = (200, 200, 200) # 蛇的颜色
- BLACK = (0, 0, 0) # 网格线颜色
- RED = (200, 30, 30) # 红色,GAME OVER 的字体颜色
- BGCOLOR = (40, 40, 60) # 背景色
-
-
- def print_text(screen, font, x, y, text, fcolor=(255, 255, 255)):
- imgText = font.render(text, True, fcolor)
- screen.blit(imgText, (x, y))
-
-
- # 初始化蛇
- def init_snake():
- snake = deque()
- snake.append((2, SCOPE_Y[0]))
- snake.append((1, SCOPE_Y[0]))
- snake.append((0, SCOPE_Y[0]))
- return snake
-
-
- def create_food(snake):
- food_x = random.randint(SCOPE_X[0], SCOPE_X[1])
- food_y = random.randint(SCOPE_Y[0], SCOPE_Y[1])
- while (food_x, food_y) in snake:
- # 如果食物出现在蛇身上,则重来
- food_x = random.randint(SCOPE_X[0], SCOPE_X[1])
- food_y = random.randint(SCOPE_Y[0], SCOPE_Y[1])
- return food_x, food_y
-
-
- def get_food_style():
- return FOOD_STYLE_LIST[random.randint(0, 2)]
-
-
- def main():
- pygame.init()
- screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
- pygame.display.set_caption('贪吃蛇')
-
- font1 = pygame.font.SysFont('SimHei', 23) # 得分的字体
- font2 = pygame.font.Font(None, 55) # GAME OVER 的字体
- fwidth, fheight = font2.size('GAME OVER')
-
- # 如果蛇正在向右移动,那么快速点击向下向左,由于程序刷新没那么快,向下事件会被向左覆盖掉,导致蛇后退,直接GAME OVER
- # b 变量就是用于防止这种情况的发生
- b = True
-
- # 蛇
- snake = init_snake()
- # 食物
- food = create_food(snake)
- food_style = get_food_style()
- # 方向
- pos = (1, 0)
-
- game_over = True
- start = False # 是否开始,当start = True,game_over = True 时,才显示 GAME OVER
- score = 0 # 得分
- orispeed = 0.5 # 原始速度
- speed = orispeed
- last_move_time = None
- pause = False # 暂停
-
- while True:
- for event in pygame.event.get():
- if event.type == QUIT:
- sys.exit()
- elif event.type == KEYDOWN:
- if event.key == K_RETURN:
- if game_over:
- start = True
- game_over = False
- b = True
- snake = init_snake()
- food = create_food(snake)
- food_style = get_food_style()
- pos = (1, 0)
- # 得分
- score = 0
- last_move_time = time.time()
- elif event.key == K_SPACE:
- if not game_over:
- pause = not pause
- elif event.key in (K_w, K_UP):
- # 这个判断是为了防止蛇向上移时按了向下键,导致直接 GAME OVER
- if b and not pos[1]:
- pos = (0, -1)
- b = False
- elif event.key in (K_s, K_DOWN):
- if b and not pos[1]:
- pos = (0, 1)
- b = False
- elif event.key in (K_a, K_LEFT):
- if b and not pos[0]:
- pos = (-1, 0)
- b = False
- elif event.key in (K_d, K_RIGHT):
- if b and not pos[0]:
- pos = (1, 0)
- b = False
-
- # 填充背景色
- screen.fill(BGCOLOR)
- # 画网格线 竖线
- for x in range(SIZE, SCREEN_WIDTH, SIZE):
- pygame.draw.line(screen, BLACK, (x, SCOPE_Y[0] * SIZE), (x, SCREEN_HEIGHT), LINE_WIDTH)
- # 画网格线 横线
- for y in range(SCOPE_Y[0] * SIZE, SCREEN_HEIGHT, SIZE):
- pygame.draw.line(screen, BLACK, (0, y), (SCREEN_WIDTH, y), LINE_WIDTH)
-
- if not game_over:
- curTime = time.time()
- if curTime - last_move_time > speed:
- if not pause:
- b = True
- last_move_time = curTime
- next_s = (snake[0][0] + pos[0], snake[0][1] + pos[1])
- if next_s == food:
- # 吃到了食物
- snake.appendleft(next_s)
- score += food_style[0]
- speed = orispeed - 0.03 * (score // 100)
- food = create_food(snake)
- food_style = get_food_style()
- else:
- if SCOPE_X[0] <= next_s[0] <= SCOPE_X[1] and SCOPE_Y[0] <= next_s[1] <= SCOPE_Y[1] \
- and next_s not in snake:
- snake.appendleft(next_s)
- snake.pop()
- else:
- game_over = True
-
- # 画食物
- if not game_over:
- # 避免 GAME OVER 的时候把 GAME OVER 的字给遮住了
- pygame.draw.rect(screen, food_style[1], (food[0] * SIZE, food[1] * SIZE, SIZE, SIZE), 0)
-
- # 画蛇
- for s in snake:
- pygame.draw.rect(screen, DARK, (s[0] * SIZE + LINE_WIDTH, s[1] * SIZE + LINE_WIDTH,
- SIZE - LINE_WIDTH * 2, SIZE - LINE_WIDTH * 2), 0)
-
- print_text(screen, font1, 5, 10, f'speed: {score//100}')
- print_text(screen, font1, 105, 10, f'score: {score}')
-
- if game_over:
- if start:
- print_text(screen, font2, (SCREEN_WIDTH - fwidth) // 2, (SCREEN_HEIGHT - fheight) // 2, 'GAME OVER', RED)
-
- pygame.display.update()
-
-
- if __name__ == '__main__':
- main()
-
复制代码
|